Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial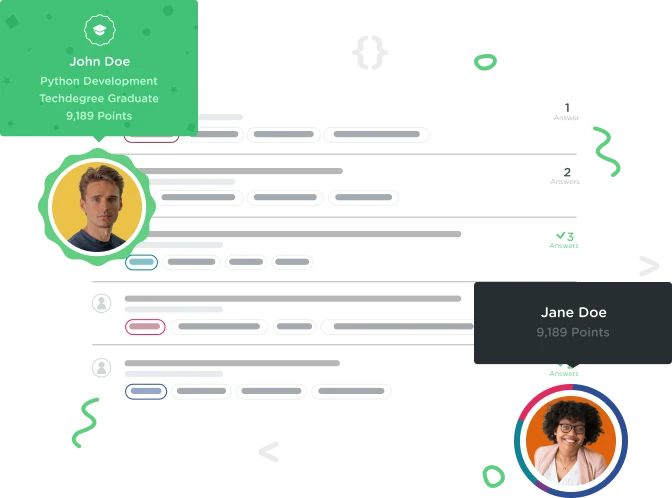

shawn khah
15,082 Pointscan't solve challenge 2 of 2
Challenge Task 2 of 2
Use the private access modifier to make it so that classes other than Frog can get the value of NumFliesEaten but canβt set it. Important: In each task of this code challenge, the code you write should be added to the code from the previous task. Restart Preview Get Help Check Work Frog.cs
1 namespace Treehouse.CodeChallenges 2 { 3 public class Frog 4 { 5 public int NumFliesEaten { get; set; } 6 } 7 }
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int NumFliesEaten { get; set; }
}
}
1 Answer

Daniel W
1,341 PointsHi!
It looks like you're having some trouble understanding the private/public properties. Public means that other classes have access to the function or property. Private means that only the class in which the function or property lives in, has access to it.
From the first task we have the following code:
public int NumFliesEaten { get; set; }
Which means NumFliesEaten can both be get and it can also be set. This also means any class can set or get the NumFliesEaten property, as it is public.
However our task is to:
"Use the private access modifier to make it so that classes other than Frog can get the value of NumFliesEaten but canβt set it. "
In other words, this means that we need to make sure that other classes can get the value, but can't set it. As you can see, NumFliesEaten has two shorthanded "functions" which are geting the value and setting the value, denoted within the curly braces.
Whenever we use NumFliesEaten = 3, we are setting or writing the value. Similarly, when we use NumFliesEaten somewhere in our code, we are reading the value.
So how do we make sure that other classes can READ the value but not WRITE to it?
Well, by changing the scope of the "function" of set. That is, we need to hide it from all the other classes, so that when other classes can not attempt to do NumFliesEaten = 3.
public int NumFliesEaten { get; private set; }
This code should make sure that other classes can read/get the property, but since the setter is private, only the Frog class can change its value!
Hope this helps.
shawn khah
15,082 Pointsshawn khah
15,082 Pointsthank's Daniel