Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial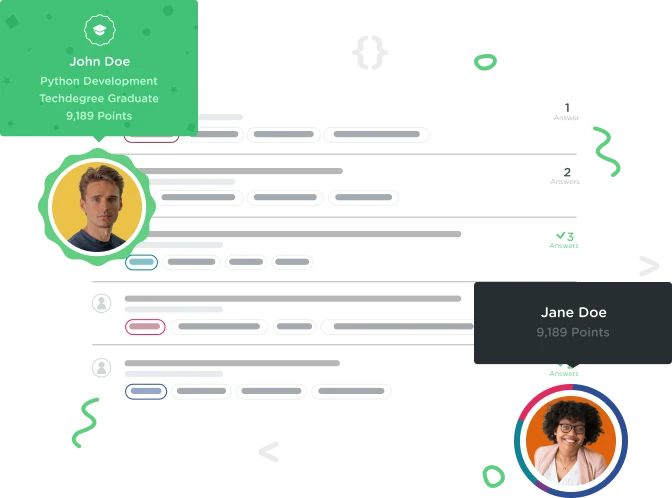

Pierce Mulligan
1,106 PointsCan't solve my compiler error.
Where is my code failing?
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
} while(!isFullyCharged()) {
mBarsCount = MAX_ENERGY_BARS;
}
}
3 Answers

andi mitre
Treehouse Guest TeacherHey Pierce,
So your syntax error is that you have a while loop after closing the isFullyCharged() method.
However, the exercise is to change the charge() method by utilizing the isFullyCharged() mehtod.
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while(!isFullyCharged()){
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
So you use the while loop with the ! to check when the gokart is not fully charged and in that case you want to charge the gokart so you increment the mBarsCount variable.
Hope that helps!
Cheers

Pierce Mulligan
1,106 PointsThat makes sense, but now I am experiencing a compiler error, but when I click preview there's no text saying where or what the error is.

andi mitre
Treehouse Guest TeacherIf you copy and delete the code it should pass. At least, it passed for me.
Thanks @piercemulligan

Pierce Mulligan
1,106 PointsI got it working on my own, thanks again.

Hayes McCardell II
643 Points public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
} while(!isFullyCharged()) {
mBarsCount = MAX_ENERGY_BARS;
}
The problem is that you are returning a value and then closing the function with a curly brace, and then adding the while loop outside of a function.
The instructions ask for the while loop using the !isFullyCharged, which looks good. But it needs to be inside the function block. The instructions also ask for the mBars to be incremented (added to) during each run through the while loop.
You can increment a value by adding something to it. Such as:
while(!full){
gas = gas + 1;
}
or
while(!full){
++gas;
}
Hopefully this helps you along the way without giving you the code ;)

Hayes McCardell II
643 PointsAlso, a return stops the code right away and exits the current code block.
Any remaining code in that function will not be executed. So make sure you do operations before you return a value.
That being said, a function can return more than one value if there is decision logic.
if (customerTall){
return large;
}
if(customerShort){
return small;
}
Pierce Mulligan
1,106 PointsPierce Mulligan
1,106 PointsI am trying to use a while loop to charge the kart when it is not fully charged "!isFullyCharged".