Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial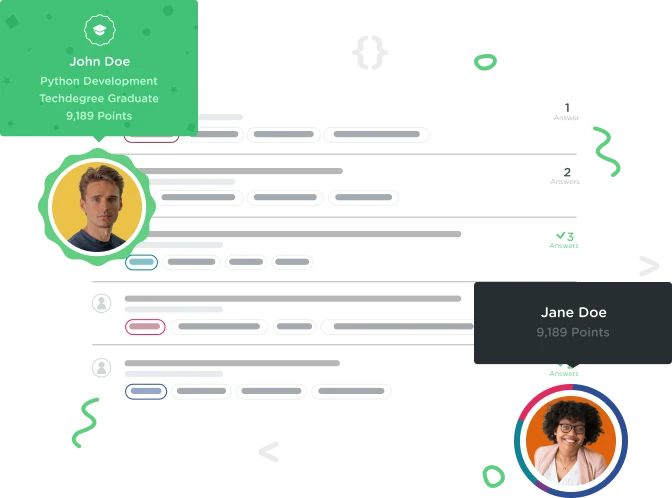
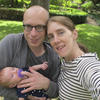
Gordon Cameron
6,600 PointsCan't solve Task 2 of 3, final code challenge, Object-Oriented Python/Instant Objects (RaceCar class exercise)
Don't know why this code isn't passing. Thanks in advance.
class RaceCar:
def __init__(self, color, laps=0, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= (self.length * 0.125)
self.laps += 1
9 Answers
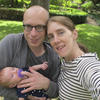
Gordon Cameron
6,600 PointsUnfortunately, with that alteration made, I still get 'bummer, try again.' Thanks anyway.
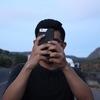
Marc Martinez
12,909 PointsIn your init method, you need to put self.laps = laps That's how I got it to work
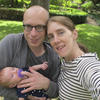
Gordon Cameron
6,600 PointsThanks, but you're critiquing Gerald's code, not mine. I always had a comma after laps=0. Doesn't work, and the 'bummer' tip gives no hints as to what's going wrong.
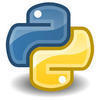
Gerald Wells
12,763 PointsCheck your indentation, I fixed The syntax error.
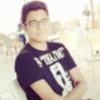
hamdi ismail
Courses Plus Student 8,927 Pointsthis should work
''' class RaceCar:
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= (length * .125)
self.laps += 1
'''
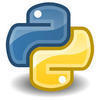
Gerald Wells
12,763 PointsYou do not call self on a method variable.
class RaceCar:
def __init__(self,color,fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for (k,v) in kwargs.items():
setattr(self,k,v)
def run_lap(self,length):
self.fuel_remaining -= length*0.125
self.laps += 1

Paul Bentham
24,090 PointsThere's a missing comma after laps=0.

TaChyla Murray
Courses Plus Student 147 PointsI encountered the same error as Gordon. It came down to the order in which I listed my arguments to the init method and the order I assigned them to the attribute on the instance using "self".
The following code fails:
class RaceCar:
def __init__(self, color, laps=0, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.laps += 1
self.fuel_remaining -= length * 0.125
The following code passes:
class RaceCar:
def __init__(self, color, fuel_remaining, laps = 0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.laps += 1
self.fuel_remaining -= length * 0.125

ashleyjorgensen
1,408 PointsIF YOU ARE COPYING AND PASTING THESE SOLUTIONS STOP AND READ. I got fed up with the problem because I thought I was doing it wrong so I started copying and pasting these solutions. Majority of the solutions above are correct. This was not a code issue for me, this was a website issue. Go through your code and backspace each line from the front of the line to the left side of the screen. Then hit 4 spaces (equals 1 tab) to push the code back over. Some spots in your code will require 8 spaces. I found that there was some weird spacing that I could not see that was preventing this from being correct even though my code was correct. I knew the code was right because I ran it on my computer so it had to be an issue with Treehouse.

Taig Mac Carthy
8,139 Pointsclass RaceCar:
def __init__(self,color,fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self,length):
self.fuel_remaining -= length*0.125
self.laps += 1
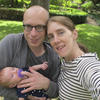
Gordon Cameron
6,600 PointsHmm, the below code passed. It seems that the exercise was happier when laps was defined outside of init
Thanks for the contributions, guys.
class RaceCar:
laps=0
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= (length * .125)
self.laps += 1

Paul Bentham
24,090 PointsThat code passed because you were no longer putting "self.length" in your run_lap method as per Gerald's comment. It didn't have anything to do with laps being defined outside of the init -> I think you have to put it back in there in the next exercise.
Paul Bentham
24,090 PointsPaul Bentham
24,090 PointsTry it with the comma after laps=0