Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial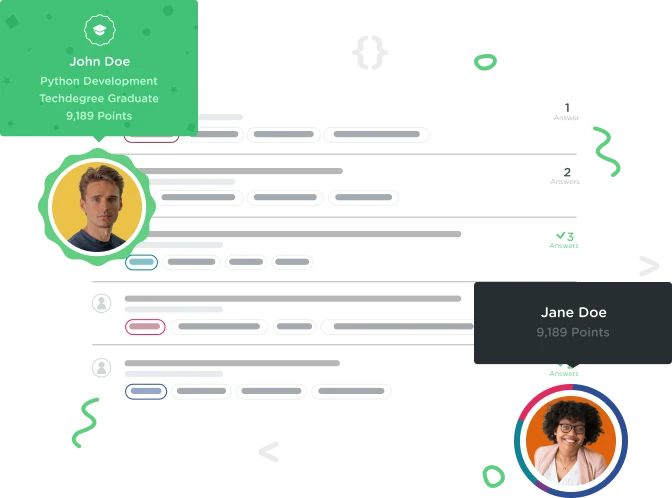

Calvin Liem
3,599 Pointscan't solve the challenge
Let's get in some practice creating a class. Declare a class named Shape with a variable property named numberOfSides of type Int.
Remember that with classes you are required to write an initializer method.
Once you have a class definition, create an instance and assign it to a constant named someShape.
so i my code is
class Shape {
var numberOfSides: Int
init(numberOfSides: Int) {
self.numberOfSides = numberOfSides
}
}
let someShape = numberOfSides
this code won't work can someone explain to me the answer
6 Answers
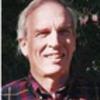
jcorum
71,830 PointsCalvin, your class is fine! You just didn't use it. Try this for the last line:
let someShape = Shape(numberOfSides: 3)
Here you set the constant someShape to be a Shape object, one with 3 sides.
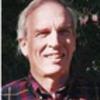
jcorum
71,830 PointsMartina, what I've found is that the instructions for some challenges are done well, but those for others aren't. Some challenges seem to have been "class-room tested", to make sure they are understandable by students. Others clearly have not. In some cases instructors have clarified the instructions when they see that students find them confusing. But some instructors seem not to care.
I'm a bit puzzled, though, why you responded this way for this challenge, as it seems to be one of the more straight-forward ones. Of course, everyone gets hung up on different things -- I know I have!
So let's go through step by step.
(1) Declare a class named Shape Start with the keyword class, then the name, capitalized, then an opening and closing curly brace:
class Shape { }
(2) *with a variable property named numberOfSides of type Int: * Start with the var keyword, then the name of the property, camelCase with lower case first letter, then, since you are not assigning a value, explicitly indicate the type, as Swift will not be able to infer it from the value:
class Shape {
var numberOfSides: Int
}
(3) write an initializer method As the instructions remind you, this is a class, and does not have a default initializer, as do structs, so you need to write it. Initializers must make sure that all instance properties have values. Since their is one such property, it needs to have one parameter, and then it needs to assign the value passed in with that parameter to the numberOfSides property. To disambiguate between the parameter name and the property name you use the keyword self followed by a dot:
class Shape {
var numberOfSides: Int
init(numberOfSides: Int) {
self.numberOfSides = numberOfSides
}
}
(4) create an instance and assign it to a constant named someShape Start with the keyword let, then add the name of the constant followed by the assignment operator, then the name of the class followed by the name of the parameter and the value that you want to pass to the class as the value of the property:
let someShape = Shape(numberOfSides: 6)
Hope this helps.
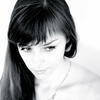
Martina Reiter
17,654 PointsIs it just me or are the instructions of challenges really not clear? it's always hard for me to understand exactly whet i have to do

Ciaran Wood
Courses Plus Student 6,787 PointsI'm finding the same problem!
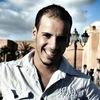
mohd zahrani
iOS Development Techdegree Student 3,013 PointsMe too!

martonvelczenbach
2,229 PointsSame here.

Oscar G
2,647 PointsThis worked for me:
class Shape {
var numberOfSides: Int = 2
}
let someShape = Shape()

Amir BenArtzi
897 PointsThis worked for me, In my case - class 'Shape' was lower cased once I upper cased Shape it passed the challenge
// Enter your code below
class Shape{
var numberOfSides: Int
init (numberOfSides: Int){
self.numberOfSides = numberOfSides
}
}
let someShape = Shape(numberOfSides: 3)

Cristina Rosales
10,929 PointsIt's the whole "Let's phrase this like we're trying to answer a question in Jeopardy" thing that threw me.
Calvin Liem
3,599 PointsCalvin Liem
3,599 Pointsomg i never see pasan use that or maybe im not pay attention to it i even try
let someShape = numberOfSides: 5
my bad thanks for the reply