Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial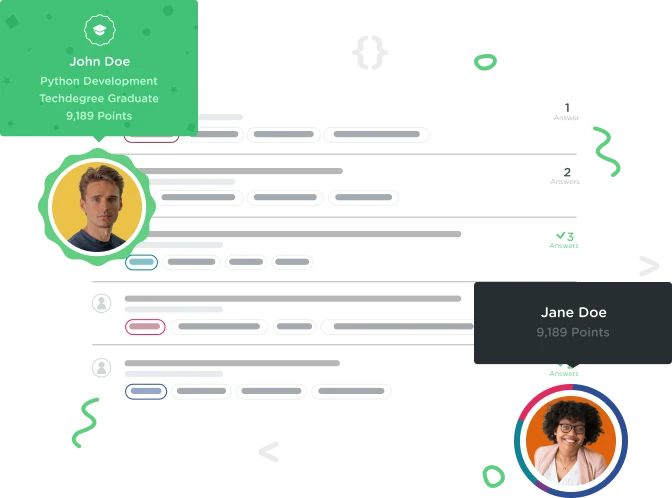
Alaa Moussa
Courses Plus Student 535 PointsCan't solve this !!
Which method below gets called first when MainActivity is interrupted by something like a phone call? 'onPause()' or 'onResume()'? In the correct one, add the call to the corresponding method in the Activity parent. Add either 'super.onPause();' or 'super.onResume();', but not both.
package com.example;
import android.os.Bundle;
import android.view.View;
import android.hardware.Sensor;
import android.hardware.SensorManager;
public class MainActivity extends Activity {
public static String aString;
private SensorManager mSensorManager;
private Sensor mAccelerometer;
private ShakeDetector mShakeDetector;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
aString = "This variable is set AFTER the 'otherString' variable in Activity";
mSensorManager = (SensorManager) getSystemService(SENSOR_SERVICE);
mAccelerometer = mSensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
mShakeDetector = new ShakeDetector();
}
public void onPause() {
super.onPause();
mSensorManager.unregisterListener(mShakeDetector);
}
public void onResume() {
super.onResume();
mSensorManager.registerListener(mShakeDetector, mAccelerometer, SensorManager.SENSOR_DELAY_UI);
}
}
2 Answers

Ernest Grzybowski
Treehouse Project ReviewerThe android activity lifecycle is very important to understand, and luckily there is a lot of documentation about it.
http://developer.android.com/guide/components/activities.html
You should try to add Log.d("","");
messages in your overridden methods! It's a fun and simple project that will really help you understand the lifecycle.
Example:
@Override
protected void onStop() {
Log.d("My Tag", "onStop() HIT!");
super.onStop();
}
@Override
protected void onResume() {
Log.d("My Tag", "onResume() HIT!");
super.onResume();
}
Try to override all of the activity methods:
onCreate()
onStart()
onResume()
onPause()
onStop()
onDestroy()
- etc
Then give yourself (or your emulator) a phone call!

Rajesh Mule
2,125 Pointspublic void onPause() { super.onPause(); mSensorManager.unregisterListener(mShakeDetector);
public void onResume() {
super.onResume();
mSensorManager.registerListener(mShakeDetector, mAccelerometer, SensorManager.SENSOR_DELAY_UI);
}
Alaa Moussa
Courses Plus Student 535 PointsAlaa Moussa
Courses Plus Student 535 PointsThank u so much for replying but i found the solution, i was so tired and i couldn't understand the question and i found the solution after understanding good the question and its Removing super.onResume(); so the app can Pause :D
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherGlad you got it! The intent of this question is to make you think about which method needs to be overridden. If it's confusing I'll look into changing the wording. :)
Alaa Moussa
Courses Plus Student 535 PointsAlaa Moussa
Courses Plus Student 535 PointsThank you so much Mr.Ben i am so happy to get a reply from you, and i would like to email you about some problems that I am facing like when and how can I be capable of doing an app using my ideas easily without watching back the courses. Its my pleasure to be educated in Tree House with you Mr.Ben
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherIf possible, please post your questions here in the Forum as you also get the benefit of multiple opinions, and the discussions may be helpful to others in the future. :) However, I'm happy to chat about stuff (time-permitting!), so you can email me at ben@teamtreehouse.com or follow me on Twitter (@benjakuben) and I'll try to answer things promptly.