Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial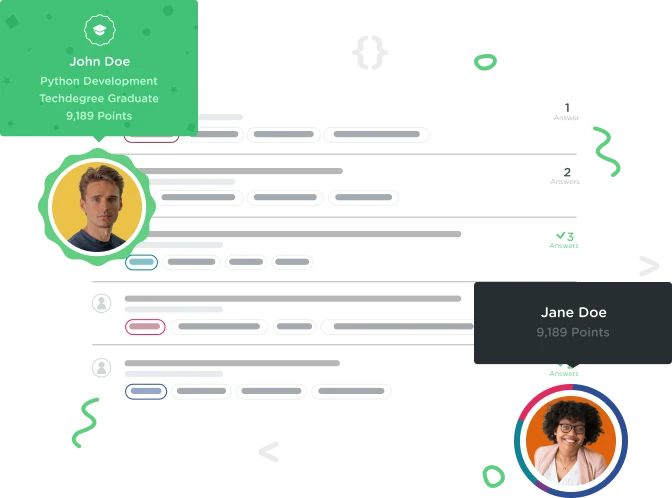

Ramy Elsaraf
7,633 PointsCant Solve this
Create a method named WordsWithCountGreaterThan that takes an integer as a parameter. The method should return a dictionary of all of the words that have a count greater than the parameter passed in. The dictionary should contain the word and the word's count.
using System.Collections.Generic;
namespace Treehouse.CodeChallenges { public class LexicalAnalysis { public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
if (WordCount.ContainsKey(word))
{
WordCount[word]++;
}
else
{
WordCount.Add(word, 1);
}
}
}
}
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
if (WordCount.ContainsKey(word))
{
WordCount[word]++;
}
else
{
WordCount.Add(word, 1);
}
}
public void WordsWithCountGreaterThan (int num){
if (WordCount > num) {
WordCount.Add(num);
}
return WordCount;
}
}
}
3 Answers

andren
28,558 PointsThere are a number of issue with your code. First of all the method is meant to return a Dictionary so it can't have a return type of void. Secondly since a Dictionary is a collection of data you can't compare it directly to an integer like you are doing in your if statement. Thirdly you are not meant to return or add to the dictionary that already exists, but to create a new one to hold the words that have a greater count.
Specifically the challenge is to:
return a dictionary of all of the words that have a count greater than the parameter passed in. The dictionary should contain the word and the word's count.
To complete that challenge you basically have to do four things:
Create a new dictionary to hold words that have a count greater than the given number
Loop though the individual words in the WordCount Dictionary and compare them to the number passed into the method.
Add the word and the word count to the new list if the word count happens to be greater than the one passed into the method.
Return the new dictionary you just created.
I would recommend that you go back and take a second look at the Dictionary Keys and Values video that proceeded this challenge as they show off how to do most of what I describe above in that video.
I would also recommend that you try the challenge again, and try to follow the instructions I laid out above. But if you are still struggling with solving the challenge then I have posted the solution for you here, along with comments about what I'm doing in the solution.
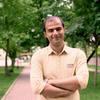
S.Amir Nahravan
4,008 Pointspublic Dictionary<string, int> WordsWithCountGreaterThan(int param) { Dictionary<string, int> result = new Dictionary<string, int>(); foreach(var item in WordCount) { if (item.Value > param) { result.Add(item.Key, item.Value); } }
return result;
}

Kevin Gates
15,053 PointsThis works. Let me know if you have any questions:
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
try
{
WordCount.Add(word, 1);
}
catch
{
WordCount[word] +=1;
}
}
public Dictionary<string,int> WordsWithCountGreaterThan(int count)
{
var resultSet = new Dictionary<string,int>();
foreach(KeyValuePair<string, int> entry in WordCount)
{
if ( entry.Value > count)
{
resultSet.Add(entry.Key,entry.Value);
}
}
return resultSet;
}
}
}