Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial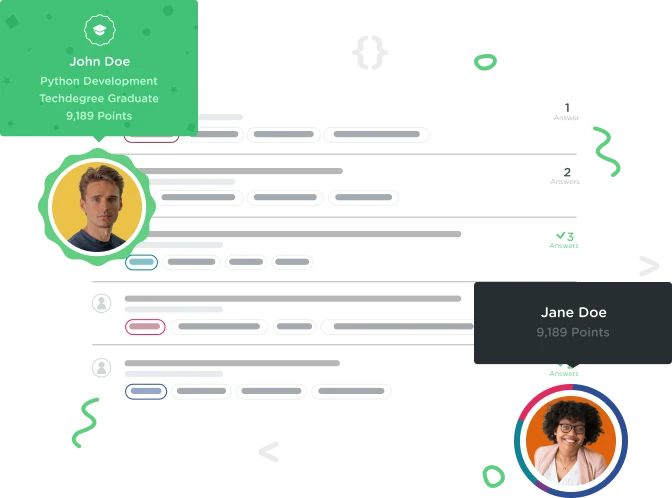

ali aboutaleb
4,025 PointsCant solve this.. anyone can help??
im having a hard time with this task
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
class Car: Vehicle {
var numberOfSeats: Int
init(withDoors doors: Int, andWheels wheels: Int, andSeats seats: Int){
self.numberOfSeats = seats
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4, andSeats: 4)
2 Answers

Heidi Puk Hermann
33,366 PointsHi Ali,
you have implemented this part of the task wrong : "Your task is to create a subclass of Vehicle, named Car, that adds an additional stored property numberOfSeats of type Int with a default value of 4."
you are asked to give a default value of 4 seats to the car class, which means that it should not be in the initializer. Your code should look like this:
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int = 4
override init(withDoors doors: Int, andWheels wheels: Int){
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4)

Heidi Puk Hermann
33,366 Pointsin that case your initializer would look like this:
class Car: Vehicle {
var numberOfSeats: Int
override init(withDoors doors: Int, andWheels wheels: Int, andSeats seats: Int){
super.init(withDoors: doors, andWheels: wheels)
self.numberOfSeats = seats
}
}
When the variable has been given an initial value, you do not need to include it in the initializer, since it is all ready initialized.
Hope it makes sence :)
sugabelly
2,524 Pointssugabelly
2,524 PointsI have a question. Based on your answer, how would you write this code if in the subclass, there was no initial value specified?
i.e. instead of the Car having a default numberOfSeats of 4, it's just of type Int. In that case, how would you initialise that in the code you wrote above? Do you use init? If so, how?