Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial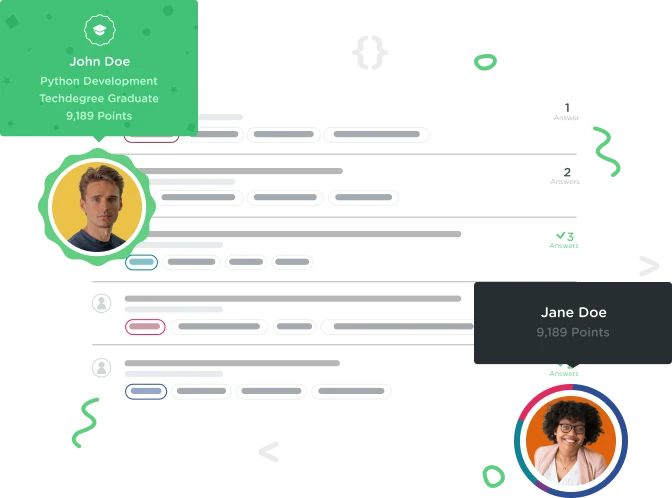
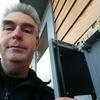
Remco de Wilde
9,864 PointsCan't solve VideoGameRepository task 1
Help
How to I get a mathing type to return?
using Treehouse.Models;
using System.Collections.Generic;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
public VideoGame GetVideoGames()
{
List <VideoGame> videoGameToReturn = new List<VideoGame>();
foreach (var videoAdd in _videoGames)
{
videoGameToReturn.Add (videoAdd);
}
return videoGameToReturn;
}
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
4 Answers

Jon Wood
9,884 PointsIn this line: private static VideoGame[] _videoGames = new VideoGame[]
it looks like you're creating a static method plus instantiating an array of VideoGame
types.
You're actually very close! First it wants you to create a GetVideoGames
method. The rest of the code is using an array, so we'll do that. Here's a skeleton method you can use:
public VideoGame[] GetVideoGames()
{
}
The next task is similar in terms of the method signature. Instead of returning an array, we want to return a single VideoGame
whose id
matches a VideoGame
from our array.
public VideoGame GetVideoGame(int id)
{
}
That should get you going, but let me know if you need more help!
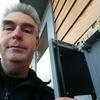
Remco de Wilde
9,864 PointsChanged the code to:
public class VideoGamesRepository
{
public VideoGame[] GetVideoGames()
{
int numberOfGames=_videoGames.Length;
int counter=1;
var videoGameToReturn = new VideoGame[numberOfGames];
foreach (var videoAdd in _videoGames)
{
videoGameToReturn[counter] = videoAdd;
counter++;
if (counter == numberOfGames)
{
break;
}
}
return videoGameToReturn;
}
but still get a error, but compiler is not complaining
Bummer! Are you returning the '_videoGames' static field from the 'GetVideoGames' method?

Jon Wood
9,884 PointsI don't think you need to do all that in that method. I think it just wants return _videoGames;
in it.
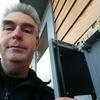
Remco de Wilde
9,864 PointsThats it :S
public VideoGame[] GetVideoGames() { return _videoGames; }
But was my way to long method working?

Jon Wood
9,884 PointsIt looks like it may work, but just not needed. :)
The _videoGames
field already gets set with the collection:
private static VideoGame[] _videoGames = new VideoGame[]
{
...
}
I was actually mistaken earlier when I mentioned that part. I didn't realize it was given to you and that it just sets the private field to a hard coded collection. Sorry about that!
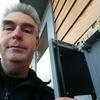
Remco de Wilde
9,864 PointsNo worry. Happy you could help me.
And trying to be a programmer and think in sollutions :D
Finished the second task :D
Thanks a lot.

Jon Wood
9,884 PointsAwesome! Glad you got it going! :)