Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial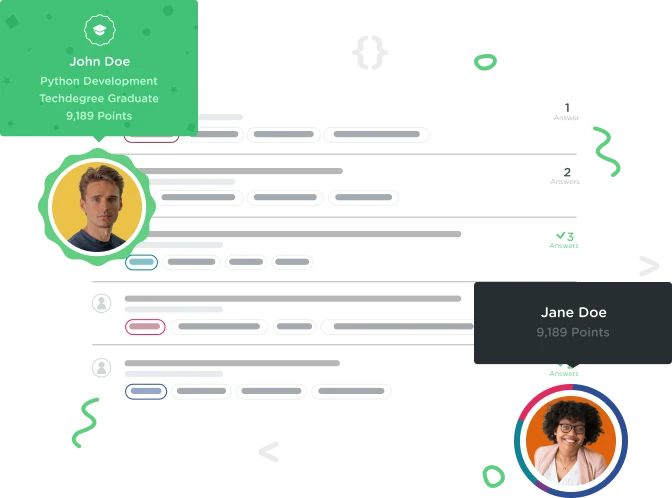

Odane Williams
6,692 PointsCan't spot why the for loop isn't appending to the list.
The script supposed to iterate through word and append each individual letter in to my_list. Then after that all the vowels are removed, and then the word returned as a whole string using the .join method.
def disemvowel(word):
word_list = []
word.lower()
for letter in word:
word_list.append(letter)
word_list.remove("a")
word_list.remove("e")
word_list.remove("i")
word_list.remove("o")
word_list.remove("u")
word = ''.join(word_list)
return word
1 Answer

Riki Montgomery
9,803 PointsYour loop is getting to word_list.remove("a") and then stopping, because word_list is empty. You can't remove something that's not there.
Odane Williams
6,692 PointsOdane Williams
6,692 PointsHow is it getting to it if it's not in the same block?
Riki Montgomery
9,803 PointsRiki Montgomery
9,803 PointsThis will help you see what's happening: http://www.pythontutor.com/
I don't know python very well, but that will help you understand how you wrote your function wrong and how you might fix it.
You'll notice that word.lower() doesn't seem to be doing anything either.
Riki Montgomery
9,803 PointsRiki Montgomery
9,803 PointsI misspoke. word_list is not empty. It's just that "a" isn't in there, so it stops the function at word_list.remove("a"). I'm not sure why. Try out pythontutor and mess around with different ways of writing this.
Riki Montgomery
9,803 PointsRiki Montgomery
9,803 PointsThis works in http://www.pythontutor.com but it breaks when you add the rest of the characters to be removed.