Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial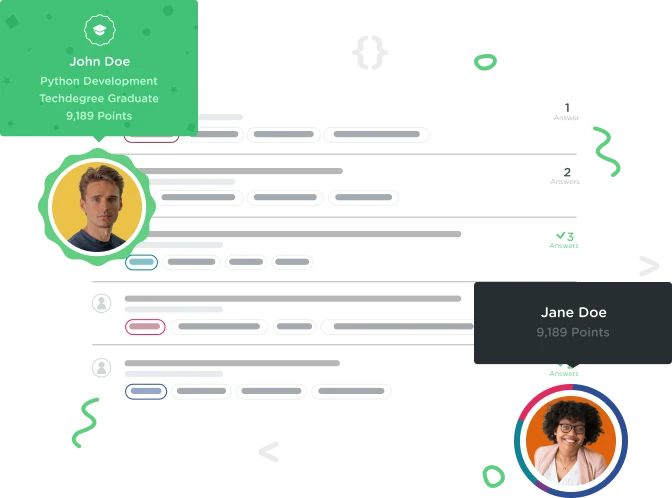

Igor Shvetsov
5,716 PointsCan't understand pointers with objects
It's all clear with primitives. But I'm a little bit confused with objects pointers. -(void) someMethod { NSString *originalString = @"string"; [self changeString:originalString]; NSLog(@"original string: %@", originalString); }
- (void)changeString:(NSString *)myString { myString = @"new string"; NSLog(@"changed string: %@", myString); }
The output: changed string: new string original string: string
I have several questions: 1) Why when we increment i we use *: *x = *x+1; But when we use NSString we don't use *: myString = @"new string"; ? 2) Why originalString hasn't changed if we passed variable by reference: (NSString *)myString ?
1 Answer
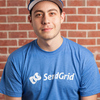
Dylan Shine
17,565 PointsAlright, so in Objective-C objects are created on the Heap rather than on the Stack. Think of the Stack as containing all your primitive variables like integers. At memory level, an Objective-C object is simply a chuck of code configured in a particular way that can be created and destroyed at will. When we instantiate an Objective-C object, what were actually doing is creating a Stack object that "points" to a heap object containing your object.
int a = 1 // Stack Object
NSString *string = @"String" // Stack Object that points to a Heap object, hence the pointer
If you would like to learn more check out this article: https://mikeash.com/pyblog/friday-qa-2010-01-15-stack-and-heap-objects-in-objective-c.html
In terms of *x = *x + 1, that code shouldn't run. You only refer to the variable as a pointer during instantiation. Secondly, you would never increment an Objective-C object with an integer int a = 1
a = a + 1 // Allowed
NSNumber *a = @1
a = a + 1 // Not allowed...cant add object of type NSNumber with int
I hope that helps.