Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial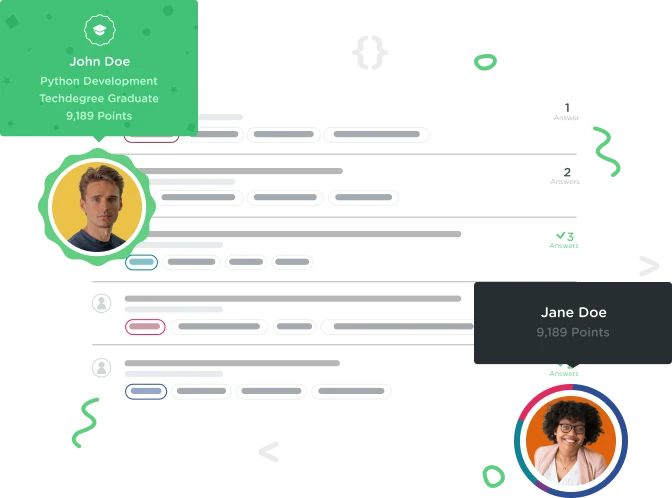

Ram Shyam
Front End Web Development Techdegree Student 85 Pointscant understand reduce proprty of an array
cant understand reduce property of an array please can someone help me i cant even understand from mdn docs please
3 Answers
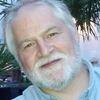
Jeff Muday
Treehouse Moderator 28,720 PointsThis is a good question to ask as it is a concept somewhat integral to array/data handling in all modern languages. "reduce" is part of the "array operations family" along with its sister functions "map" and "shuffle". You will see this repeated in Python, Ruby, Java, and other modern languages.
https://en.wikipedia.org/wiki/MapReduce
This reduce method allows a way to programmatically apply a function to each member of the array and return a new value. The value returned is a computationally "reduced" version of the array. You can use very specific callback functions inside the parenthesis of the reduce.
The callback can have any name, but MUST include an accumlator var as its first parameter (this name comes from old-school computing assembly language where an accumulator was a number inside the CPU that held onto the value of the last computation) simliar to the displayed number in the calculator. The accumulator variable's name can be any valid JavaScript variable name. And the callback MUST include a variable that will have the current value of an array element as its second variable. The last is an optional initial value to load into the accumulator at the start of the computation. (note: there are additional complexities, but not going into that)
function myCallBack( accumulator, currentValue, optInitialValue) {
return someComputedValue;
}
Here's an example -- please read the comments which explain three very simple "reduce" operations.
// here's our array
var myArray = [1,2,3,4,5];
// here's a function that just returns 0 no matter what the array has
// a very dumb function, but easy to understand.
function alwaysZero(accumulator, currentValue) {
return 0;
}
// here's a function to apply that simply returns a sum
// of the accumulator and a the currentValue of the array.
function sum( accumulator, currentValue) {
return accumulator + currentValue;
}
// here's another function
function max(accumulator, currentValue) {
// this function compares the accumulator to the currentValue and
// returns the one that is greater.
if (currentValue > accumulator) {
return currentValue;
}
return accumulator;
}
// returns zero, that's all I can do.
console.log(myArray.reduce(alwaysZero));
// returns a sum of the array
// 1 + 2 + 3 + 4 + 5 = 15
console.log(myArray.reduce(sum));
// returns the max value in the array
// 5
console.log(myArray.reduce(max));
In reality, do you need to use reduce? No, you could easily code these steps as stand alone functions with loops and computations and a return statement. But, the reduce
function makes it easy to write code that closely conforms to mathematical operations on big data sets.
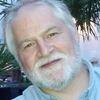
Jeff Muday
Treehouse Moderator 28,720 PointsAn accumulator is a value held internally during the operation and receives the computed value at each step. I will simulate it the sum
operation below.
Historically speaking the idea of accumulator takes place from stepwise computations:
https://en.wikipedia.org/wiki/Accumulator_(computing)
Below is code that uses an accumulator variable. It should be pretty clear if you have gotten this far in JavaScript. And learning JavaScript is a great choice since JavaScript is arguably the most popular programming language in the world!
// this is a sum function with the accumulator
var accumulator = 0;
var myArray = [1,2,3,4,5]
console.log("Beginning the reduce operation");
for (var i = 0; i < myArray.length; i++) {
console.log("** iteration: " + (i+1) + " **");
// currentValue gets the value at the current element in myArray
currentValue = myArray[i];
console.log("The currentValue = " + currentValue);
// below, the accumulator takes the value of itself added with the currentValue
accumulator = accumulator + currentValue;
console.log("The accumulator =" + accumulator);
}
console.log("Ending the reduce operation");
console.log("The completed reduce accumulator returns the value ==> " + accumulator);
Beginning the reduce operation
** iteration: 1 **
The currentValue = 1
The accumulator =1
** iteration: 2 **
The currentValue = 2
The accumulator =3
** iteration: 3 **
The currentValue = 3
The accumulator =6
** iteration: 4 **
The currentValue = 4
The accumulator =10
** iteration: 5 **
The currentValue = 5
The accumulator =15
Ending the reduce operation
The completed reduce accumulator returns the value ==> 15

Ram Shyam
Front End Web Development Techdegree Student 85 Pointsthanks bro got a very good help from u
Ram Shyam
Front End Web Development Techdegree Student 85 PointsRam Shyam
Front End Web Development Techdegree Student 85 Pointsyeah bro thanks for ur explaination but i dont understand what is accumulator and currentvalue thats the big problem bro please tell