Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial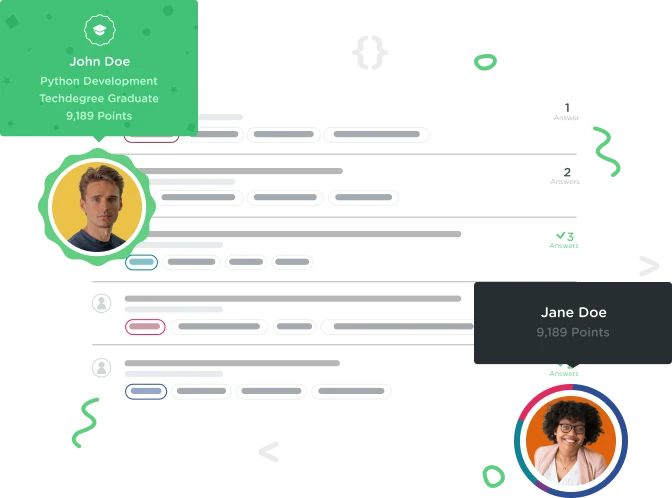

Madina Begumova
2,930 PointsCan't understand the issue. Please help
my code is not accepted
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
override init() {
super.init()
}
override func move(direction: String){
switch direction{
case "Up" : location.y += 1
case "Down" : location.y -= 1
case "Left" : location.x -= 1
case "Right" : location.x += 1
default : break
}
}
}
let player = Robot()
player.location = Point(x: 2, y: 2)
player.move(direction: "Up")
print(player.location.y)
2 Answers
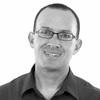
David Papandrew
8,386 PointsThe problem is that the parent Class, Machine, has a function "move" with an external name of "_"
Your subclass function should have the same external name AND you need to remove the parameter name from the player.move statement.
Here's the tweaked code. With comments marking the spots you need to address.
Should pass once you make these changes.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
override init() {
super.init()
}
override func move(_ direction: String){ // Add the underscore
switch direction{
case "Up" : location.y += 1
case "Down" : location.y -= 1
case "Left" : location.x -= 1
case "Right" : location.x += 1
default : break
}
}
}
let player = Robot()
player.location = Point(x: 2, y: 2)
player.move("Up") // Underscore in the func means no param name, just pass argument
print(player.location.y)

Madina Begumova
2,930 Pointsthank you so much, David! I was able to fix it myself as well :)