Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial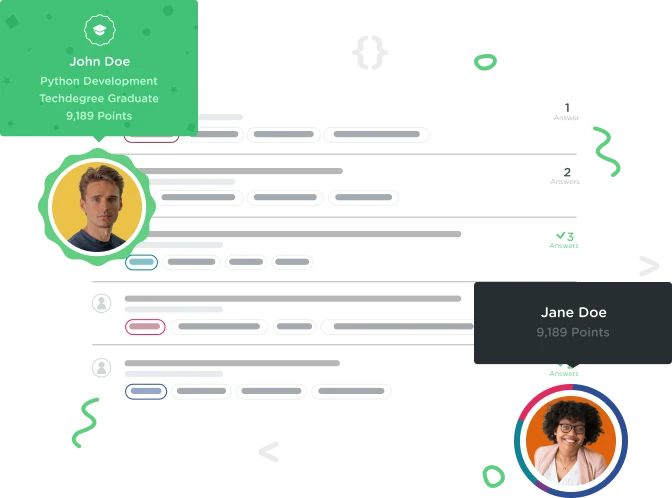
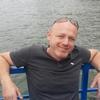
Michael Konstantinovsky
991 PointsCan't understand what is wrong?
Hi everybody, Need some help please, everything looks good and working on anaconda. Thanks!
def stringcases(string):
reverse = []
[reverse.append(index) for index in list(string)[::-1]]
return tuple(string.lower()),tuple(string.upper()),tuple(string.capitalize()),tuple(reverse)
9 Answers
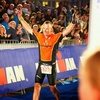
Steve Hunter
57,712 PointsThink about what this line is doing:
[reverse.append(index) for index in string[::-1]]
Confusing brackets aside, what you're doing is reversing a string then working through, from its first element to its last, and appending each character to the reverse
variable. Like this:
reverse = []
string = "treehouse"
backwards = string[::-1] # backwards now holds 'esuoheert'
for character in backwards: # iterate through the string one-by-one
reverse.append(character) # add each one into reverse
So you start with a string holding treehouse
you then reverse it, esuoheert
and the iterate through that one character at a time and assign each individual character into reverse
, e
then s
then u
then o
then h
then e
then e
then r
then t
. See the point here? You can just use the method you've used to reverse the string in the return statement, string[::-1]
.
Make sense?
Steve.
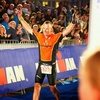
Steve Hunter
57,712 PointsHi Michael,
There's a method for three of these tasks; just the reverse one needs a little more thought.
Your main issue is what you are returning. A tuple is a series of values separated by a comma and surrounded with parentheses. You don't need to use the word tuple
on any of these.
You've correctly identified the lower()
and upper()
methods. You might want to check through the documentation for sting methods to see what capitalise
does and why this isn't what we need here. There is another method, though - keep scrolling down.
For the last one; maybe try iterating backwards over the word. You've used [::-1]
, quite correctly, in your code. There's no need to convert string
to a list or to create a new variable to hold it. Just use [::-1]
on the string
directly.
Let me know how you get on.
Steve.
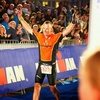
Steve Hunter
57,712 PointsHi Michael,
If you look at the question, the returned tuple is supposed to return an uppercase string first. You haven't done that. So, switch the first two elements around.
Also, you don't need the reverse
variable. It doesn't add much. Just return the expression that reverses a list:
def stringcases(string):
return (string.upper(), string.lower(), string.title(), string[::-1])
Steve.
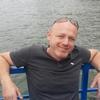
Michael Konstantinovsky
991 PointsHi Steve, Thank you so much for quick reply and help. I didn't imagine that an order in the return line, might have significance! Thank you!
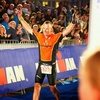
Steve Hunter
57,712 PointsYeah - the order can be critical in some cases. It's about what the person calling the method is expecting to receive back from it.
Anyway, glad you got it fixed and good luck with the rest of the course. Shout if you need help!
Steve.
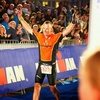
Steve Hunter
57,712 PointsHi Michael,
Could you have a read through my initial answer. This says to omit the word tuple
- you won't need that at all in this challenge.
Further, there's no need to create the additional variable, reverse
at all. The inside of the method can be a single line and still be clear.
If you work through the points in my above answer, you should be guided to the solution.
Steve.
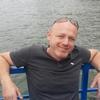
Michael Konstantinovsky
991 PointsHi Steve, Thanks for quick reply, I changed to "title()" (you are right!) and removed conversion to list (good input), but still I getting this error: "Got the wrong string(s) for: Uppercase, Lowercase, Reversed, Titlecase" Maybe I don't understand what they want to do here? Thanks
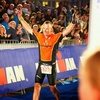
Steve Hunter
57,712 PointsHi Michael,
Let's have a look at your code and I'll talk you through a fix.
Steve.
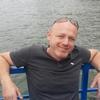
Michael Konstantinovsky
991 PointsHi Yoong, Thank you for your help, but I think, I have a problem with understanding the question
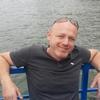
Michael Konstantinovsky
991 Pointsdef stringcases(string):
reverse = []
[reverse.append(index) for index in string[::-1]]
return tuple(string.lower()),tuple(string.upper()),tuple(string.title()),tuple(reverse)
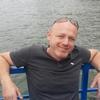
Michael Konstantinovsky
991 PointsHey Steve, It makes sense!!! Thanks a lot, I understand my mistake with reversing, and your method is perfect. Also I removed 'tuple' and checked my function with/without prentices in return line and keep receiving following error: " Bummer! Got the wrong string(s) for: Uppercase, Lowercase" Do you have any idea why? Thank a lot for your help!!! This is my code now:
def stringcases(string):
reverse = string[::-1]
return (string.lower(),string.upper(),string.title(),reverse)
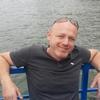
Michael Konstantinovsky
991 PointsAnd by the way when I checking it in anaconda with "type()" command, I getting "tuple" as an answer. Thanks again!
Yoong Kang Lim
5,273 PointsYoong Kang Lim
5,273 PointsBy "something wrong" you mean you're seeing tuples ('h', 'e', 'l', 'l', 'o') instead of strings "hello"?
Don't use
tuple(string.lower())
then.