Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial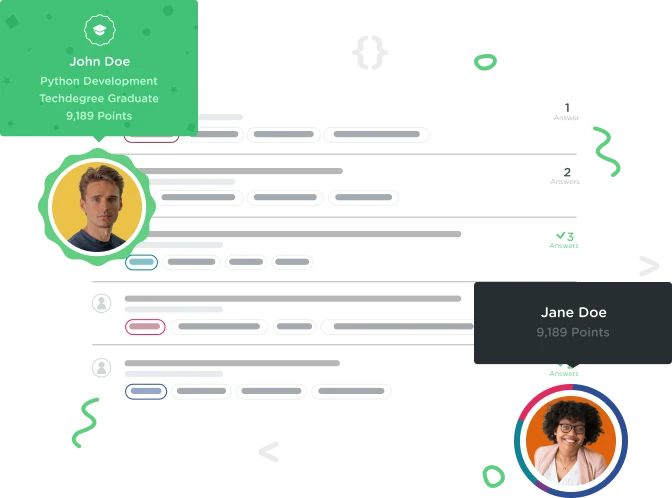

David Zhang
946 PointsCan't use regEx here?
I'd finished this challenge using for loop.
However I want to try to use regEx to simplify the code, but it seems my regEx code was somehow wrong. I can't figure it out myself, thanks for any help.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
private String normalizeDiscountCode(String code) {
if (!code.matches("/^[a-zA-Z\\$]*$/")) {
throw new IllegalArgumentException("Invalid discount code");
}
return code.toUpperCase();
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer
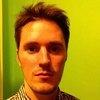
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsInteresting. It seems like the pattern that it want's shouldn't include the //
characters we normally wrap regex with. Maybe that's how regexes work in Java, I dunno.
private String normalizeDiscountCode(String code) {
if (!code.matches("^[a-zA-Z\\$]*$")) {
throw new IllegalArgumentException("Invalid discount code");
}
return code.toUpperCase();
}
David Zhang
946 PointsDavid Zhang
946 PointsI just tried whatever the patterns are(even like ^[a-z]*$), there always prompt message "Bummer: java.lang.IllegalArgumentException: Invalid discount code (Look around Order.java line 25)". I don't know what went wrong...
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsDo all those patterns include the // characters? I think that's the problem. Your original pattern worked when I removed the slashes. Basically,
code.matches
was returning false because there was a problem with the pattern, which then had us enter theif
block and threw the exception.David Zhang
946 PointsDavid Zhang
946 PointsDamn, I'd just removed the open and close / sign in my code, and it all worked no matter with or without double escape sign. I will remember this lesson.
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsSorry that's what I meant, not the escape signs.
David Zhang
946 PointsDavid Zhang
946 PointsThanks for your time and patience. I think my reading needs improving. Lmao that miscommunicating.