Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial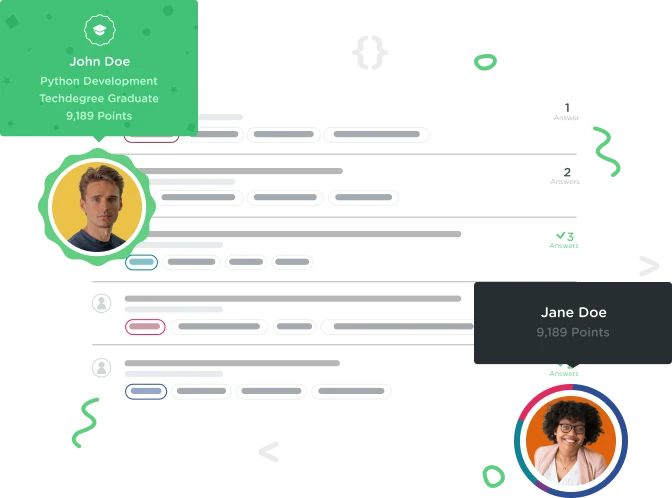
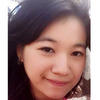
Cherie T
3,429 PointsCan't use .remove() to remove the list from inside of messy_list. Why?
Hi there. Going through Challenge Task 2 of 2 in Python Collections on Lists.
For some reason, my code can remove the bool and str but not list... only when using messy_list.remove[1,2,3] does it remove the list. Why is that?
Thanks!
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.insert(0,messy_list.pop(3))
for item in messy_list:
if item is not int:
messy_list.remove(item)
messy_list.remove[1,2,3]
1 Answer
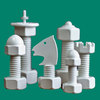
Steven Parker
231,275 PointsRemoving items from an iterable while it is controlling a loop can cause other items in the loop to be skipped over. To prevent this, use a copy of the iterable to control the loop.
Also, I wouldn't expect "item is not int
" to be a viable test. Did you mean to use the "type" function?
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsHey Steven, Can you expand on this? How would you use a for loop to get rid of certain data 'types'. I can't seem to get it to work. This is what I have.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou'd want to put the "type" function on the item. And you can use the identity operator here:
if type(item) is not int:
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsHey Steven, Thanks for the help. When I made the adjustment to the code it deleted the bool and the string but left the list. Why is this? Also, is there a rule of thumb on when to use identity operators?
Thanks again for your help!
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou didn't show the code where "copy_list" is created. I'm suspecting it might not be a copy but just another reference to the list and the skipping over might still be taking place.
A good example use of the identity operator would be checking my assumption (before the loop):
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsHere is my full code
I actually haven't tried this for the treehouse challenge, I'm using my Geany editor and printing the output to my console. I'm positive that it isn't every other one though- it is printing all of the integers that are in consecutive order. The output I'm getting is [2, 3 ,1, [1, 2, 3]]
********You're right, I wasn't copying the list correctly**********************
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYup, I guessed it, that's just a reference to the same list. You can confirm it with the little test program in the previous example. To get a copy of the list you can do this.
copy_list = messy_list.copy() # or messy_list[:]
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsAwesome! Thanks for your help!