Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial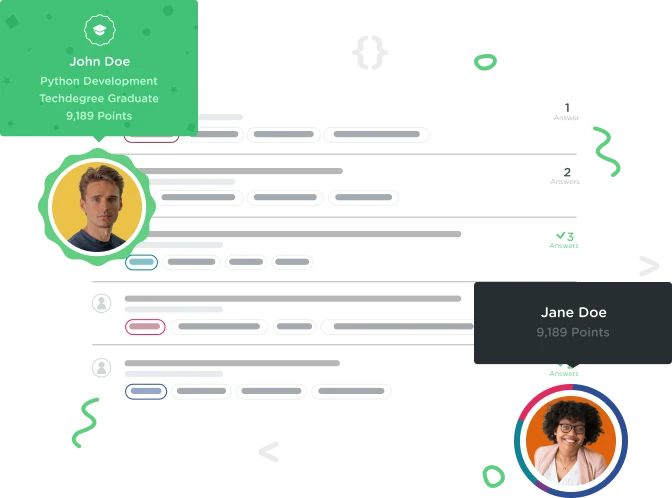

Mark Riddell
15,970 PointsCan't view Actors Table in DB browser
The newly created actors will print to the console, but when i refresh DB browser, only the movies db shows up without any actors. Will post code in comments.

Mark Riddell
15,970 Pointsindex.js
const Sequelize = require('sequelize');
const sequelize = new Sequelize({
dialect: 'sqlite',
storage: 'movies.db',
logging: false
});
const db = {
sequelize,
Sequelize,
models: {},
};
db.models.Movie = require('./models/movie.js')(sequelize);
db.models.Actor = require('./models/actor.js')(sequelize);
module.exports = db;

Mark Riddell
15,970 Pointsactor.js
const Sequelize = require('sequelize');
module.exports = (sequelize) => {
class Actor extends Sequelize.Model {}
Actor.init({
firstName: {
type: Sequelize.STRING,
allowNull: false,
validate: {
notNull: {
msg: 'Please provide a value for "firstName"',
},
notEmpty: {
msg: 'Please provide a value for "firstName"',
}
},
},
lastName: {
type: Sequelize.STRING,
allowNull: false,
validate: {
notNull: {
msg: 'Please provide a value for "lastName"',
},
notEmpty: {
msg: 'Please provide a value for "lastName"',
}
},
},
},{sequelize});
return Actor;
};
2 Answers
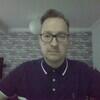
Brian Wright
6,770 PointsPromise.all() is going to return an array of promises in the order they were entered. You need to handle the returned promises before you can display the data.
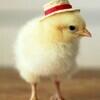
Mark Westerweel
Full Stack JavaScript Techdegree Graduate 22,378 PointsI think I know what's up:
Go to the "Browse Data" tab next tot he "Database Structure" tab.
Switch Movies table to Actor table. You should see it now (and no longer the movies). You created another table, remember?
You see them both in the console, since you specifically printed them to both (node runs all console.log's and prints them). In the DB browser, this isn't the case (another interface).
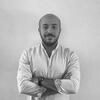
Yazan Abbas
5,299 Pointsthanks!
Mark Riddell
15,970 PointsMark Riddell
15,970 PointsApp.js