Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial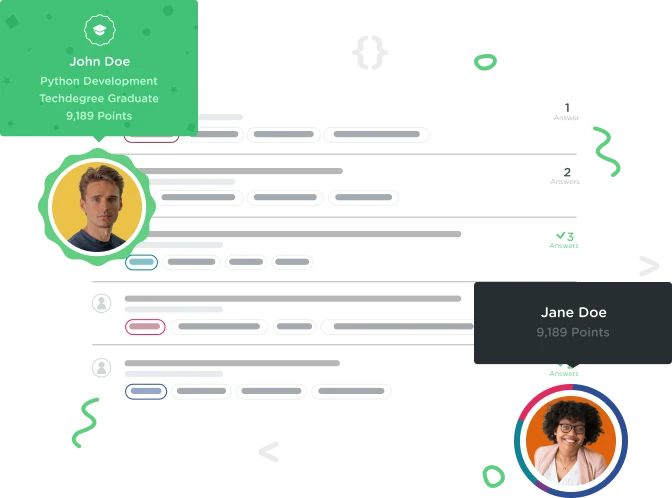
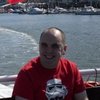
Sean Flanagan
33,235 PointsCan't work out what's wrong
Hi there. My code generates a syntax error.
import random
# safely create an integer
# limit number of guesses
# "too high" message
# "too low" message
# play again
def game():
# generate random number between 1 and 100
secret_num = random.randint(1, 10)
num_of_guesses = []
while len(num_of_guesses) < 5:
try:
# get number guess from player
guess = int(input("Guess a whole number between 1 and 10. "))
except ValueError:
print("{} isn't a number.".format(guess))
else:
# compare guess to secret number
if guess == secret_num:
print("Well done! My number was: {}".format(secret_num))
break
elif guess < secret_num:
print("Higher than {}".format(guess))
else:
print("Lower than {}".format(guess))
num_of_guesses.append(guess)
else:
print("Bad luck! My number was {}".format(secret_num))
play_again = input("Do you want to play again? Y/n"
if play_again.lower() != "n": # Error on this line
game()
else:
print("Thanks for playing. Bye!")
Can someone please tell me what's wrong?
Thanks
Sean
5 Answers

artkay
5,401 PointsHi Sean,
Yeah, it's okay. It will run the game should user decide to go ahead and play again.
The game()
will just make it work for the first script run.
Hope this helps.
Art.
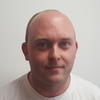
Perry Marquer
11,243 PointsYour input does not have a closing parenthesis. )

artkay
5,401 PointsJust as mentioned above - the problem is here:
play_again = input("Do you want to play again? Y/n"
# ^^^
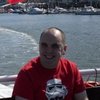
Sean Flanagan
33,235 PointsHi guys. Thanks for your efforts to help.
import random
# safely create an integer
# limit number of guesses
# "too high" message
# "too low" message
# play again
def game():
# generate random number between 1 and 10
secret_num = random.randint(1, 10)
num_of_guesses = []
while len(num_of_guesses) < 5:
try:
# get number guess from player
guess = int(input("Guess a whole number between 1 and 10. "))
except ValueError:
print("{} isn't a number.".format(guess))
else:
# compare guess to secret number
if guess == secret_num:
print("Well done! My number was: {}".format(secret_num))
break
elif guess < secret_num:
print("Higher than {}".format(guess))
else:
print("Lower than {}".format(guess))
num_of_guesses.append(guess)
else:
print("Bad luck! My number was {}".format(secret_num))
play_again = input("Do you want to play again? Y/n ") # Closing parenthesis
if play_again.lower() != 'n':
game()
else:
print("Thanks for playing. Bye!")
Now the program won't run at all. No error message or anything. I'm not sure what to do.
Sean

artkay
5,401 PointsSean, you need to run it. The script just defining the function.
Try adding this to the end of the script:
game()
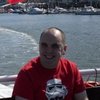
Sean Flanagan
33,235 PointsHi artkay.
So I should add game()
to the end of the script, even though it's already under the line if play_again.lower() != 'n':
?
Thanks.
Sean
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi Art.
I did as you said and the program works now. I can't understand why Kenneth didn't need to add
game()
twice, like I did, though when I unindented line 31 and everything below it and pressed Enter, the cursor was over-indented (too far to the right).But I do appreciate your assistance.
Thank you
Sean :-)