Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial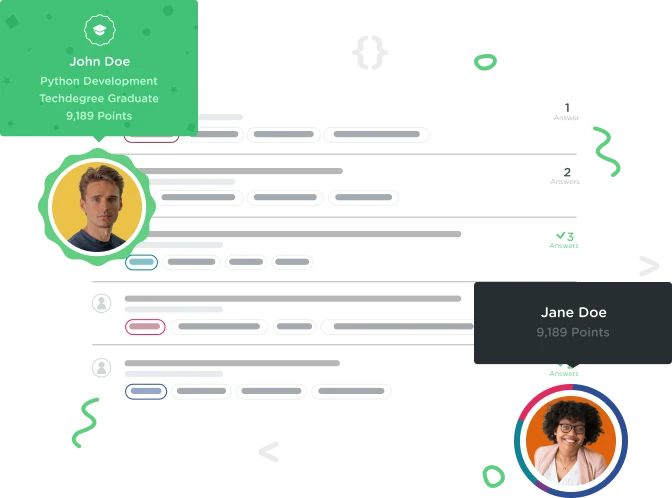

Björn Norén
9,569 PointsCanvas Animation Project
Hello dear Treehouse Community! Thanks for being awesome!
I'm working with animtations in canvas, and have accidentally created a "Saurons Eyeball". I intented to create a long rotating object, like a clock, but I don't know how to erase the before-existing object. I tried to create a second "white" object spinning a little laiter but it only got weird.
So, anyone who knows how to fix this, and can show me or tell me how? Thanks! (And how do I do to not making the code look like a mess when I do my questions?)
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
body {
margin: 40px; background:#666;
}
#my_canvas {
background:#fff; border:#000 1px solid;
}
</style>
<script>
function draw(){
var canvas = document.getElementById("my_canvas");
var ctx = canvas.getContext("2d");
ctx.translate(ctx.canvas.width/2, ctx.canvas.height/2);
var degree = (Math.PI / 180) * 1;
function spinnBall(){
ctx.rotate(degree);
ctx.beginPath();
ctx.strokeStyle="black";
ctx.moveTo(0,0);
ctx.lineTo(200,0);
ctx.stroke();
ctx.rotate(degree - degree);
ctx.beginPath();
ctx.strokeStyle="white";
ctx.moveTo(0,0);
ctx.lineTo(200,0);
ctx.stroke();
}
var iv = setInterval(spinnBall, 10);
}
window.onload = draw;
</script>
</head>
<body>
<canvas id="my_canvas" width="500" height="500">
</canvas>
</body>
</html>
1 Answer
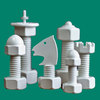
Steven Parker
230,274 PointsI'm a beginner with canvas myself, but I figured I'd give it a try.
We can start by agreeing that "degree - degree" will always be 0, right? So the 2nd rotate does nothing and can be removed.
I then tried moving the erase (white) section before the rotate and draw (black), but I still saw the same Moire pattern. I'm guessing this is just some kind of aliasing error due to the calculations.
So I had the idea to erase a wider line (and a pixel longer) than was drawn. So I tried this:
function spinnBall() {
ctx.beginPath();
ctx.strokeStyle = "white";
ctx.moveTo(0, 0);
ctx.lineTo(201, 0);
ctx.lineWidth = 3;
ctx.stroke();
ctx.rotate(degree);
ctx.beginPath();
ctx.strokeStyle = "black";
ctx.moveTo(0, 0);
ctx.lineTo(200, 0);
ctx.lineWidth = 1;
ctx.stroke();
}
And .. that seems to work!
Oh, to keep your code clean, you blockquote it as I did above. Follow the instructions in the Markdown Cheatsheet link below the answer section, and remember to skip a blank line before your quoted code.
Björn Norén
9,569 PointsBjörn Norén
9,569 PointsDude! Well done figuring that out, that would have taken me forever!
Thanks for helping me out again, I appreciate it alot!
Steven Parker
230,274 PointsSteven Parker
230,274 PointsI revised my answer using a wider line instead of a rectangle. See above.