Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial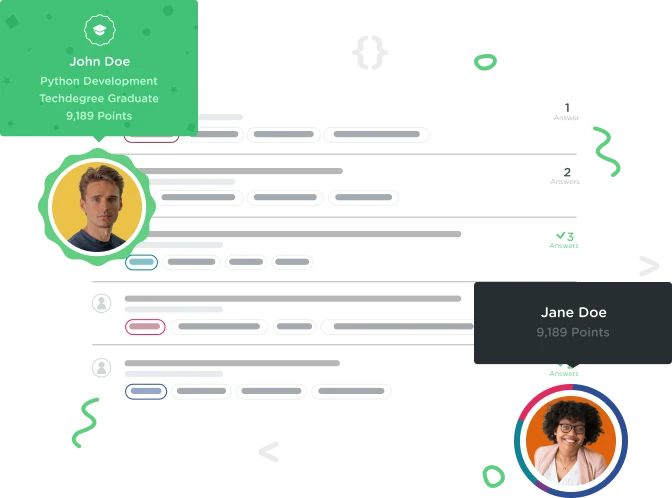

marvelousperson206
9,199 PointsCanvas not drawing
The code seems fine, but the pen won't draw. It was all going smoothly until I got up to this Part 5 video..
//Problem: No user interaction causes no change to application
//Solution: When user interacts, cause changes appropriately
var color = $(".selected").css("background-color");
var $canvas = $("canvas");
var context = $canvas[0].getContext("2d");
var lastEvent;
var mouseDown = false;
//When clicking on control list items
$(".controls").on("click", "li", function(){
//Deselect sibling elements
$(this).siblings().removeClass("selected");
//Select clicked element
$(this).addClass("selected");
//Cache current color
color = $(this).css("background-color");
});
//When new color is pressed
$("#revealColorSelect").click(function(){
//Show color select or hide the color select
changeColor();
$("#colorSelect").toggle();
});
//Update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + "," + g + "," + b + ")");
}
//When color sliders change
$("input[type=range]").change(changeColor);
//When add color is pressed
$("#addNewColor").click(function(){
//Append the color to the controls ul
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"))
$(".controls ul").append($newColor);
//Select the new color
$newColor.click();
});
//On mouse events on the canvas
$canvas.mousedown(function(e){
lastEvent = e;
mouseDown = true;
}).mousemove(function(e){
//Draw lines
if(mouseDown) {
context.beginPath();
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
context.lineTo(e.offsetX, e.offsetY);
context.strokeStyle = color;
context.stroke();
lastEvent = e;
}
}).mouseup(function(){
mouseDown = false;
});
Does anyone know what the problem is?
4 Answers

Saskia Lund
5,673 PointsHi Russell,
I copied your JavaScript code into a new .js file on my Mac. Implemented it into the canvas project and it works just fine , when I open it in Google Chrome.
Maybe a Firefox issue?
EDIT: not maybe. it is a firefox issue. -> https://bugzilla.mozilla.org/show_bug.cgi?id=69787#c37 Someone explained why offsetX and Y don't work in FF like so "The above code works perfectly on any webkit based browser. While moving the mouse it draws points on the canvas. Unfortunately it doesn't in Firefox as its event model does not support the offsetX / Y properties." A fix to the firefox lack of offsetX and Y: http://stackoverflow.com/questions/12704686/html5-with-jquery-e-offsetx-is-undefined-in-firefox
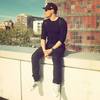
Zoltán Holik
3,401 PointsFirefox does not like offsetX and offsetY.
var x = e.offsetX == undefined ? e.layerX : e.offsetX;
var y = e.offsetY == undefined ? e.layerY : e.offsetY;
Here is an article with more info: http://www.jacklmoore.com/notes/mouse-position/

marvelousperson206
9,199 PointsThanks guys for looking into the issue! Got it sorted now, much appreciated.

William McManus
14,819 PointsHere is something strange:
I was having this exact problem. I am using Chrome to view the site, but I am writing / editing in CodeRunner, mot workspaces.
I found this question and just for fun, even though the code was exactly the same, I cut and and pasted it to Code Runner and refreshed, and it worked perfectly.
So I typed it in again in CodeRunner and once again it broke.
My question is:
Are there known glitches in CodeRunner? or is it a syntax thing. I noticed that CodeRunner wanted to autofill different selectors than we ended up using. It is really strange, has anyone had a problem like this?
marvelousperson206
9,199 Pointsmarvelousperson206
9,199 PointsI just downloaded the complete project file and tried using it through Sublime Text and still won't draw, so I'm thinking it's a browser issue? Am using the latest Firefox