Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial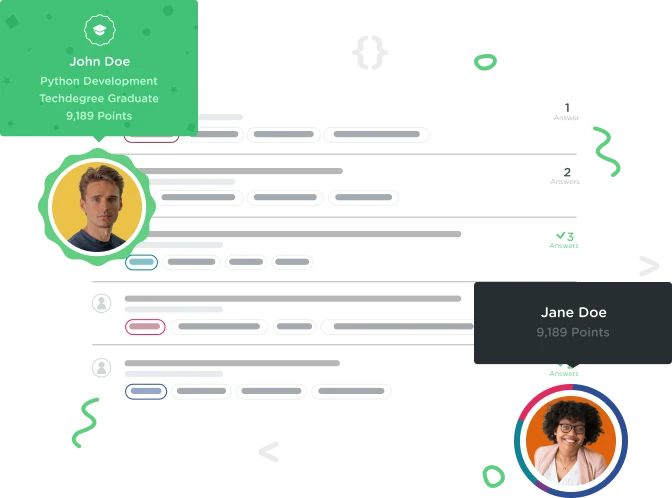
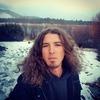
Patrick Jimosse
3,319 PointsCapitalism The Game Step 2
Really stuck. I feel like I need to add the second die. Not sure though.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size=2, die_class=D6)
@property
def doubles(self):
hand = []
if hand[0] == hand[1]:
return True
if hand[0] != hand[1]:
return False
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}

Eldin Guzin
6,010 PointsI have a follow-up question to this, why do we use if self[0] == self[1] ? why didn't we use hand[0]...? What does self mean in this example ? why didn't the first code you wrote above work ?
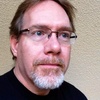
Chris Freeman
Treehouse Moderator 68,423 PointsEldin Guzin, self
refers to the current class instance. The original code does work because hand
is defined as an empty list that has none of the properties or methods found in a Hand
instance.
The method code is running βinsideβ the class. It does not have a way to reference its instance name. That is, it does not know the name of its variable label (βhandβ, in this case). βselfβ is a label that is used to point to the same thing as the unknowable variable label points to.
Post back if you need more help. Good luck!!!
1 Answer
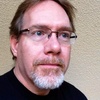
Chris Freeman
Treehouse Moderator 68,423 PointsGet job figuring it out. As an additional tip, you can replace:
if cond:
return True
else not cond:
return False
with the preferred style:
return cond
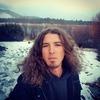
Patrick Jimosse
3,319 PointsAww, that's much more simple/beautiful. Appreciate the additional input. :D
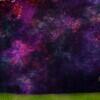
Spencer Hurrle
3,128 PointsWhy would self.dice not work the same? The dice list is created/updated as an attribute in the class through the _by_value method, isn't it?
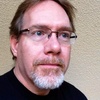
Chris Freeman
Treehouse Moderator 68,423 PointsSpencer Hurrle, in the method _by_value
, the list label dice
is a variable local to the method and is not accessible outside the method. That is, the attribute self.dice
is undefined.
Patrick Jimosse
3,319 PointsPatrick Jimosse
3,319 PointsNevermind, figure it out.