Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial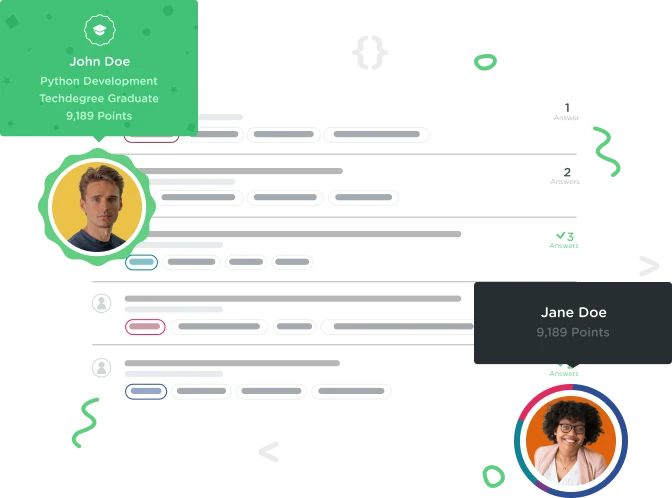

andrew falcone
8,651 PointsCapitalismHand, part 3: Is doubles supposed to return true/false while also modifying the hand if it is doubles?
Originally, doubles was supposed to return true/false depending on if you had doubles or not.
Part 3 says, that if you have doubles you now want to reroll hand effectively giving a new hand. I tried having doubles return true/false still, but also calling reroll if there are doubles and replacing self with a new capitalism hand, but the hand reverts to its original values after leaving doubles (does this have something do with self being a value type and not reference?).
Also, should reroll keep being called if a new doubles hand is rolled until a non-doubles hand is achieved?
I don't know this game, the directions are unclear, and the feedback 'Bummer: try again!' is absolutely useless.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size = 2, die_class = D6)
@classmethod
def reroll(cls):
return(cls())
@property
def doubles(self):
if max(len(self.ones), len(self.twos),len(self.threes),len(self.fours),len(self.fives),len(self.sixes)) == 2:
self = CapitalismHand.reroll()
return true
else:
return false
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
1 Answer
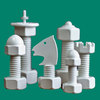
Steven Parker
229,732 PointsI'm not sure how you got to task 3, but task 2 has some issues:
- Python is case sensitive, "true" is not the same thing as "True" (same with "false" and "False")
- "doubles" should only test if doubles exist, it should not re-roll the hand
- the "reroll" method for task 3 is fine as-is, it does not need a test or a loop
andrew falcone
8,651 Pointsandrew falcone
8,651 PointsThanks, the capital letters fixed it. I noticed the lowercase thing and was pretty sure I had capitalized it both in my editor and workspace, but I guess not.
If you were to reroll the die in doubles, do you know why self doesn't get updated with the new roll?
Steven Parker
229,732 PointsSteven Parker
229,732 PointsRe-assigning "self" only changes it within the scope of the method. It doesn't have any effect on the object itself.