Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial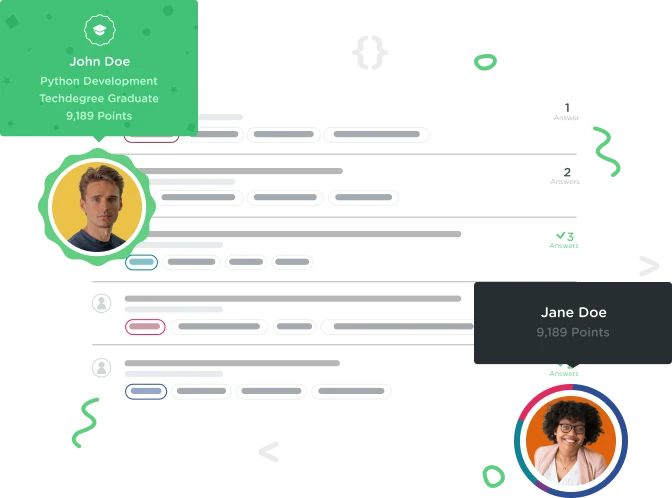

Luke Haddad
6,082 PointsCare if you give me some feedback on my solution?
//The program begins with an alert popping up greeting the user.
alert("Greetings newbies! This will just take a moment of you time if you would like to test your knowledge. Good luck!");
//This is where I put the questions and answers into a 2-Dimensional array, as well as the variables for the reward system.
var firstPlace = "Top of the class! Impressive!";
var secondPlace = "Nicely done, but not quite there yet...";
var thirdPlace = "On the right track, but need improvement.";
var points = 0;
var quesAns =
[
[
'Who was Archduke Franz Ferdinand?', 'HEIR TO THE AUSTRO-HUNGARIAN EMPIRE'
],
[
'Who was Abraham Lincoln?', 'SIXTEENTH PRESIDENT OF THE UNITED STATES'
],
[
'How many bills did F.D.R get pushed through Congress in the first one hundred days of office?', 'FIFTEEN'
],
[
'Generally speaking, how many American lives were lost in the aftermath of the Japanese attack on Pearl Harbour?', 'OVER TWO THOUSAND THREE HUNDRED'
],
[
'How many calories are found in a party-sized bottle of Sprite?', 'ONE HUNDRED AND FOURTY'
],
];
/*
This is where the user's score, the questions the user got wrong, and the right answers to those questions the user got incorrectly displays to the browser.
Also, this is where the questions are prompted to the user, and the whole test is put into a loop.
*/
var answerCorrect, answerUser;
var correctAnsIndex = [];
var wrongAnsIndex = [];
for ( var i = 0; i < quesAns.length; i += 1 ) {
answerUser = prompt( question.toUpperCase[ i ] ( 0 ) );
answerCorrect = prompt ( question[ i ] ( 1 ) );
if ( answerUser === answerCorrect )
{
alert( "Correct! The right answer is " + answerCorrect + ". " );
points += 1;
correctAnsIndex.push( i );
}
else
{
alert( "Uh-uh, incorrect! The correct answer is " + answerCorrect + ". Moving on..." );
points -= 1;
wrongAnsIndex.push( i );
}
}
document.write( "<strong>These are the questions you answered correctly: >" + correctAnsIndex.length + ". </strong><br />" );
if ( correctAnsIndex.length > 0 )
{
document.write( "These are the questions you got correct:<br />" );
for ( i = 0; i < correctAnsIndex; i += 1 )
{
document.write( questions[ correctAnsIndex [ i ] ] [ [ 0 ] ] + "<br />" );
}
else
{
document.write( "Oh no! An incorrect question! Try again?" );
}
}
document.write( "<strong>These are the questions you answered incorrectly: " + wrongAnsIndex + ". </strong><br />" );
if ( wrongAnsIndex.length > 0 )
{
document.write( "These are the questions you got incorrect:<br />" );
for ( i = 0; i < wrongAnsIndex; i += 1 ) {
document.write( ( questions[ wrongAnsIndex [ i ] ] [ [ 0 ] ] + "<br />" );
}
else
{
document.write( "Congratulations! You got all the questions correct!" );
}
}
//This is where the score is tallied up, and the user is award either first, second, or third place.
if (points === 4 || points === 5)
{
document.write (firstPlace + ". Your score is: " + points);
}
else if (points === 2 || points === 3)
{
document.write (secondPlace + ". Your score is: " + points);
}
else (points === 0 || points === 1)
{
document.write (thirdPlace + ". Your score is: " + points);
}
alert( "Thank you for participating! Farewell!" );
1 Answer

Paul Bentham
24,090 PointsWhy don't you use a "places" object and have {1:"blah blah", 2:"blah blah", 3:"blah blah"}?
Also, your if statement at the end, instead of having "points === 4 || points === 5" why not have "points > 3"?