Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial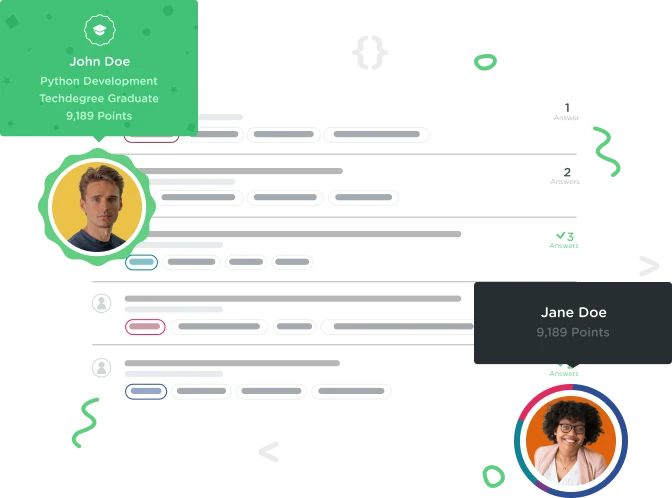
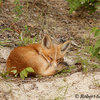
foxtails
3,655 PointsCatch illegal argument exception. Where is the problem?
Hi, can anyone tell me, where the problem is? Thank you!
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(2);
try {
kart.drive(2);
} catch (IllegalArgumentException iae) {
System.out.printf("The error was: %s.\n", iae.getMessage());
}
}
}
2 Answers

andren
28,558 PointsThe problem is that the code contains two calls to kart.drive, one that was in the code from the start and one you added inside the try...catch block. The exception raised from the second kart.drive is caught, but the first one isn't.
The point of the challenge was actually to wrap the existing call to drive in a try...catch block, you were not meant to add a new call like you have done.
To fix it just cut the try..catch block you made and paste it over the "kart.drive(2)" line in the code like this:
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(2);
} catch (IllegalArgumentException iae) {
System.out.printf("The error was: %s.\n", iae.getMessage());
}
}
}
Doing that will allow you to complete the challenge.
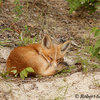
foxtails
3,655 PointsThank you very much!