Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial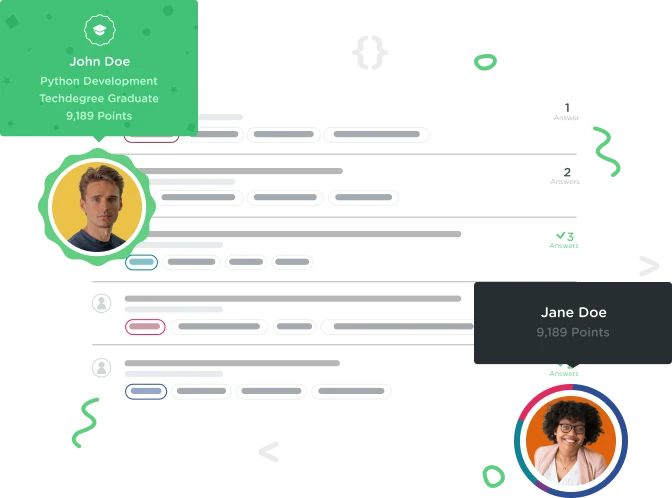
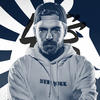
Kevin Haube
12,265 PointsCatching a JSONException, don't know why!
The class in question is below
private void getForecast() {
String apiKey = "09b2001e4b878941580a9e3460cb83e4";
double lat = 39.3711;
double lon = -76.9725;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey + "/" + lat + "," + lon;
if (isNetworkAvailable()) {
toggleRefresh();
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
alertUserOfError();
}
@Override
public void onResponse(Response response) throws IOException {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
Log.d(TAG, "JSON Response :: " + response.body().string());
try {
if (response.isSuccessful()) {
String jsonData = response.body().string();
mForecast = parseForecastDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUserOfError();
}
} catch (IOException e) {
Log.e(TAG, "IOException!! :: " + e);
alertUserOfError();
} catch (JSONException e) {
Log.e(TAG, "JSONException!! :: " + e);
alertUserOfError();
}
}
});
} else {
Toast.makeText(this, getString(R.string.network_unavailable), Toast.LENGTH_LONG).show();
}
}
Within the onResponse() inner-class, I'm being thrown a JSONException claiming that there's no value for Temperature, but when I print jsonData.body().string()
to the log, it shows that every value has the correct value. The key, lat, long, and builder for the string are all working. I can't figure out why it's catching that exception.
EDIT
Here are my getters for the current, daily, and hourly forecasts:
private Forecast parseForecastDetails(String jsonData) throws JSONException {
Forecast forecast = new Forecast();
forecast.setCurrent(getCurrentDetails(jsonData));
forecast.setHourlyForecast(getHourlyForecast(jsonData));
forecast.setDailyForecast(getDailyForecast(jsonData));
return forecast;
}
private Day[] getDailyForecast(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject daily = forecast.getJSONObject("daily");
JSONArray data = daily.getJSONArray("data");
Day[] dailies = new Day[data.length()];
for (int i = 0; i < data.length(); i++) {
JSONObject jsonDay = data.getJSONObject(i);
Day day = new Day();
day.setSummary(jsonDay.getString("summary"));
day.setTempMax(jsonDay.getDouble("temperature"));
day.setIcon(jsonDay.getString("icon"));
day.setTime(jsonDay.getLong("time"));
day.setTimezone(timezone);
dailies[i] = day;
}
return dailies;
}
private Hour[] getHourlyForecast(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject hourly = forecast.getJSONObject("hourly");
JSONArray data = hourly.getJSONArray("data");
Hour[] hours = new Hour[data.length()];
for (int i = 0; i < data.length(); i++) {
JSONObject jsonHour = data.getJSONObject(i);
Hour hour = new Hour();
hour.setSummary(jsonHour.getString("summary"));
hour.setTemp(jsonHour.getDouble("temperature"));
hour.setIcon(jsonHour.getString("icon"));
hour.setTime(jsonHour.getLong("time"));
hour.setTimezone(timezone);
hours[i] = hour;
}
return hours;
}
private Current getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject currently = forecast.getJSONObject("currently");
Current current = new Current();
current.setHumid(currently.getDouble("humidity"));
current.setTime(currently.getLong("time"));
current.setIcon(currently.getString("icon"));
current.setPrecipChance(currently.getDouble("precipProbability"));
current.setSummary(currently.getString("summary"));
current.setTemp(currently.getDouble("temperature"));
current.setTimezone(timezone);
return current;
}
2 Answers
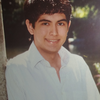
Jon Kussmann
Courses Plus Student 7,254 PointsCan you post your parseForecastDetails method?
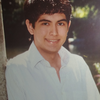
Jon Kussmann
Courses Plus Student 7,254 PointsIn your getDailyForecast method, the key for the max temperature is "temperatureMax", not "temperature".
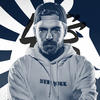
Kevin Haube
12,265 PointsThanks for catching that and squashing that bug before it happened, but I still get the same JSONException.
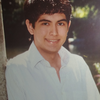
Jon Kussmann
Courses Plus Student 7,254 PointsI can't see anything wrong. I've compared your code to mine, and it looks roughly the same...
Could you humor me and move the line:
String jsonData = response.body().string();
outside of your if statement? So, right after you open your try block...
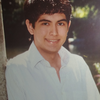
Jon Kussmann
Courses Plus Student 7,254 PointsAlso, what version of the JDK do you have installed?
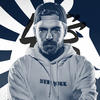
Kevin Haube
12,265 PointsI don't know why, but that actually worked...no JSONExceptions, data loads...but the whole time, it was skipping because of if(response.isSuccessful()) { ... }
so I don't know how that could have been the problem. :/
Java 1.7.0_71
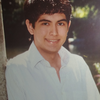
Jon Kussmann
Courses Plus Student 7,254 PointsHaha, glad that worked.
Kevin Haube
12,265 PointsKevin Haube
12,265 PointsFor further clerification, I posted that, as well as all 3 getter methods