Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial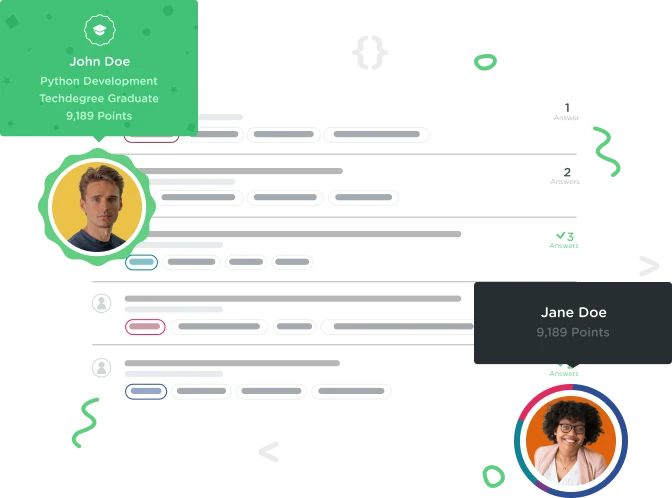

Alan Kuo
7,697 PointsCatching Exception
So I did the quiz that asks us to determine which exception will be caught, I'll paste the questions and answers below. 2 of them are confusing me, please advise me the logic and reason behind it, thank you.
Quiz Question 3 of 6 If DoSomething() throws TreehouseDefense.OutOfBoundsException, which catch clause will catch it?
try
{
DoSomething();
}
catch(TreehouseDefense.TreehouseDefenseException ex)
{
}
catch(System.Exception ex)
{
}
ANS: catch(TreehouseDefense.TreehouseDefenseException ex)
Quiz Question 5 of 6
If DoSomething() throws TreehouseDefense.TreehouseDefenseException, which catch clause will catch it?
try
{
DoSomething();
}
catch(TreehouseDefense.OutOfBoundsException ex)
{
}
ANS:
The exception won't be caught here.
1 Answer
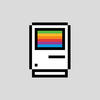
Hakim Rachidi
38,490 PointsType hierarchy
Hi Alan,
First of to clarify some things: Every Exception is of Type System.Exception.
catch(System.Exception ex) { }
This block will search for an Exception of Type System.Exception which is every Exception. Based on this we can say which exceptions a catch block will catch and which not.
An arguments or variables type must be more general then the Instance it is capturing
Object alan = new Person("Alan"); // obj is more general (is the parent class of Person) then the Person class
Person alan = new Object(); // will not work because Person Type is more specific
The same for method parameters, properties (...) and of course, catch blocks and there arguments: If the thrown Exception is more specific then the type passed to the catch block it will catch it.
try {
throw new OtherException("This exception is based on NewException which is on itself based on System.Exception");
}
catch(NewException nEx) {
// Exception will be caught here because OtherException is more specific than NewException
}
catch(Exception ex) {
// Every Exception which is not based on NewException (because those are already handled by the above block) will be handled here
}
Now back to your question.
- TreehouseDefense.OutOfBoundsException is based on TreehouseDefense.TreehouseDefenseException -> Which is why it is catch by it in question 3.
try
{
DoSomething(); // throws TreehouseDefense.OutOfBoundsException
}
catch(TreehouseDefense.TreehouseDefenseException ex)
{
}
catch(System.Exception ex)
{
}
- TreehouseDefense.TreehouseDefenseException is based on System.Exception
In question 5 the more general Exception is thrown (TreehouseDefense.TreehouseDefenseException) so the catch with the more specific type will let it pass.
It would catch it if the passed in Type is more general then the thrown Exception
try
{
DoSomething(); // throws TreehouseDefense.TreehouseDefenseException which is more specific then System.Exception
}
catch(Exception ex) // is most general Exception Type
{
}
In this and every other case the exception would be caught.
Hope this helps;
Alan Kuo
7,697 PointsAlan Kuo
7,697 PointsThank you so much Hakim Rachidi. Not only it's kind for you to answer my question but you also did it in an elegant way, thank you again!