Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial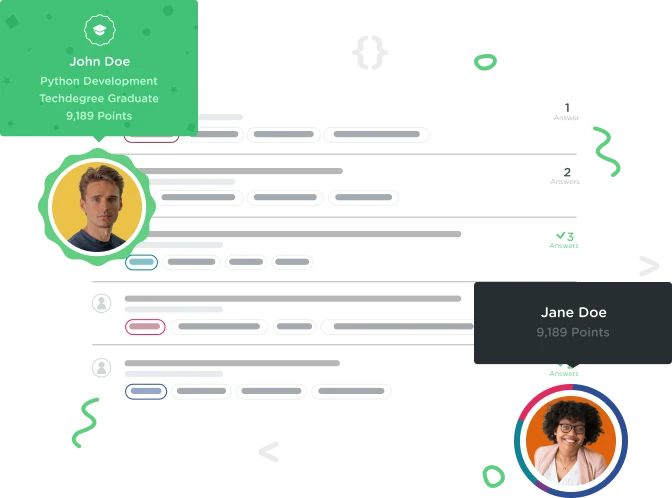

Fabio Neto da Silva
12,440 PointsChalenge = Manipulating an Array ????
Manipulating an Array Chalenge
This block of code creates an array of ice cream flavors and displays them in ascending order. The owner of the ice cream shop would like this order changed so that the flavors are displayed in descending order instead, starting with Cookie Dough first and Jalapeno So Spicy last. Leave the $flavors array itself in the same order, but modify something else in this code block to achieve that.
<html>
<body>
<?php
$flavors = array(
"Jalapeno So Spicy",
"Avocado Chocolate",
"Peppermint",
"Vanilla",
"Cake Batter",
"Cookie Dough"
);
?>
<ul>
<?php
$list_html = "";
foreach($flavors as $flavor) {
$list_html = $list_html . "<li>";
$list_html = $list_html . $flavor;
$list_html = $list_html . "</li>";
}
echo $list_html;
?>
</ul>
</body>
</html>
I really don't know where to put array_reverse()
Tried to change:
$list_html = ""; to $list_html = array_reverse($flavors);
But it doesn't work!
Help! :)
3 Answers
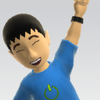
David Kaneshiro Jr.
29,247 PointsUse a different variable name for the output of the array_reverse() function, don't use $list_html. Maybe call the new array $reverse_flavors. Also make sure to call the array_reverse() function before the foreach loop.
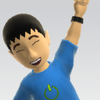
David Kaneshiro Jr.
29,247 PointsYou're welcome
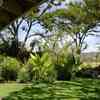
MUZ140150 Yaso
6,651 PointsThis worked for me
<html> <body> <?php
$flavors = array( "Jalapeno So Spicy", "Avocado Chocolate", "Peppermint", "Vanilla", "Cake Batter", "Cookie Dough" );
?> <ul> <?php
$list_html = "";
$reverse_flavors = array_reverse($flavors);
foreach($reverse_flavors as $flavor) {
$list_html = $list_html . "<li>";
$list_html = $list_html . $flavor;
$list_html = $list_html . "</li>";
}
echo $list_html;
?> </ul> </body> </html>
Fabio Neto da Silva
12,440 PointsFabio Neto da Silva
12,440 Pointsstill not working
What did i do wrong? :(
David Kaneshiro Jr.
29,247 PointsDavid Kaneshiro Jr.
29,247 PointsSorry, in the foreach loop change $flavors to $reverse_flavors.
Fabio Neto da Silva
12,440 PointsFabio Neto da Silva
12,440 PointsThanks David!
it worked!