Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial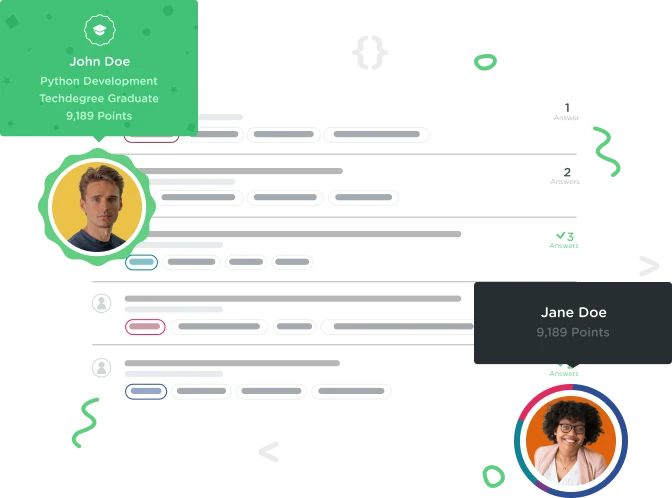

michael edmondson
4,510 PointsChallenge
Not sure what i should do with the const color square should i remove it from the event handler or put it at the top of the page and not sure what i should do with the red button and blue button. should i turn them into if statments?
const redButton = document.getElementById('redButton');
const blueButton = document.getElementById('blueButton');
redButton.addEventListener('click', (e) => {
const colorSquare = document.getElementById('colorDiv');
colorSquare.style.backgroundColor = 'red';
});
blueButton.addEventListener('click', (e) => {
const colorSquare = document.getElementById('colorDiv');
colorSquare.style.backgroundColor = 'blue';
})
div {
height: 50px;
float: left;
padding-top: 40px;
padding-left: 20px;
}
#colorDiv {
padding: 0;
width: 100px;
height: 100px;
background-color: gray;
}
button {
height: 30px;
border-radius: 10px;
}
#redButton {
background-color: #ff5959;
border-color: red;
}
#blueButton {
background-color: lightblue;
border-color: #7C9EFC;
}
1 Answer
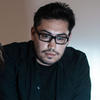
Fernando Boza
25,384 Pointsmichael edmondson you're right with your first instinct. to keep it DRY you just need to remove
const colorSquare = document.getElementById('colorDiv');
from red button eventlistner function and make it "global" so both event listeners can access and modify it.
const redButton = document.getElementById('redButton');
const blueButton = document.getElementById('blueButton');
const colorSquare = document.getElementById('colorDiv'); // moved it up here
redButton.addEventListener('click', (e) => {
colorSquare.style.backgroundColor = 'red';
});
blueButton.addEventListener('click', (e) => {
colorSquare.style.backgroundColor = 'blue';
})