Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial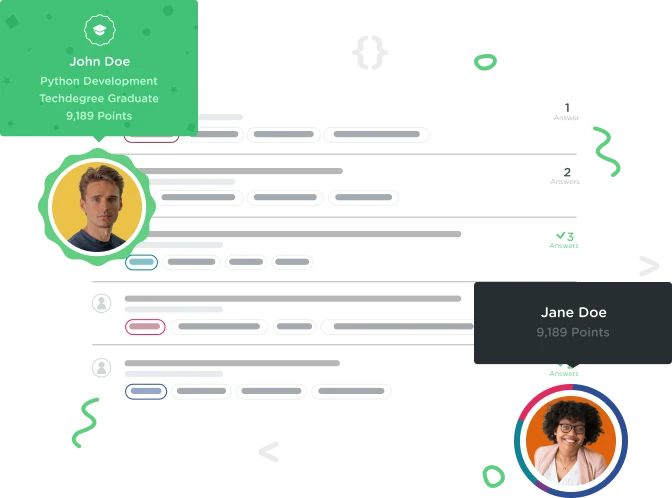

shrusha shende
1,156 Pointschallenge #2 I am just not getting what to do next
This is my code, I am not getting what am i doing wrong
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private String normalizeDiscountCode( String discountCode) {
this.discountCode = discountCode.toUpperCase();
return this.discountCode;
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
char dollar = '$';
if(!Character.isLetter(discountCode.charAt(0)) || !discountCode.contains("$") ) {
throw new IllegalArgumentException("Invalid discount code");
}
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer
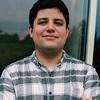
gabrieldalbuquerque
2,925 PointsHey there,
First:
- Your validation should be inside the normalizeDiscountCode() method
Second:
- You are not returning anything on your applyDiscountCode() method
Logic:
- Example.java should call applyDiscountCode() passing the codes.
- applyDiscountCode() should then call normalizeDiscountCode() passing the same code
- normalizeDiscountCode() should then process the code (validating and normalizing it) and return it in uppercase to applyDiscountCode() that would then return it to Example.java
I hope it helps.