Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial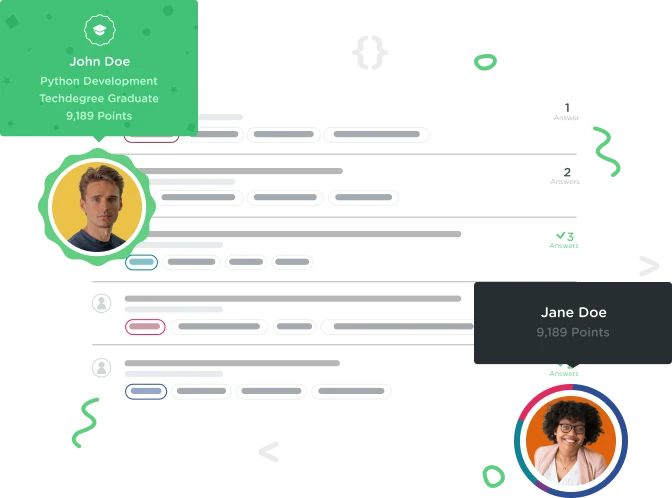

Manobhav Pandey
1,031 PointsChallenge 2 of 2 in python basics
Q- Now, make a function named summarize that also takes a list. It should return the string "The sum of X is Y.", replacing "X" with the string version of the list and "Y" with the sum total of the list.
I think the code challenges should be above reviewing what we've learnt. In task 1 it was to 'add the items of a list' which we haven't even learnt about. Can someone please explain the task here? Thanks in advance !
def add_list(list):
sum = 0
for items in list:
sum = sum + items
return(sum)
def summarize(list):
x = ''.join(str(e) for e in list)
y = 0
2 Answers

Boris Ivan Barreto
6,838 PointsHello Pandey,
Some tips for this challenge, if you need to increase or decrease the value of your variable you should use the follow: variable += something > this is equivalent to sum = sum + items.
You can also use the str() function to change the list into string.
Have a look my code
def add_list(items):
sum = 0
for item in items:
sum += item
return sum
def summarize(my_list):
return "The sum of {} is {}".format(str(my_list), add_list(my_list))
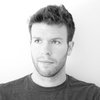
Matthew Rigdon
8,223 PointsHere is the way that I solved it, which in my opinion is simpler and uses more of the class's knowledge:
def add_list(list):
sum = 0
for items in list:
sum += items
return sum
def summarize(list):
sum = add_list(list)
return ("The sum of {0} is {1}".format(list, sum))