Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial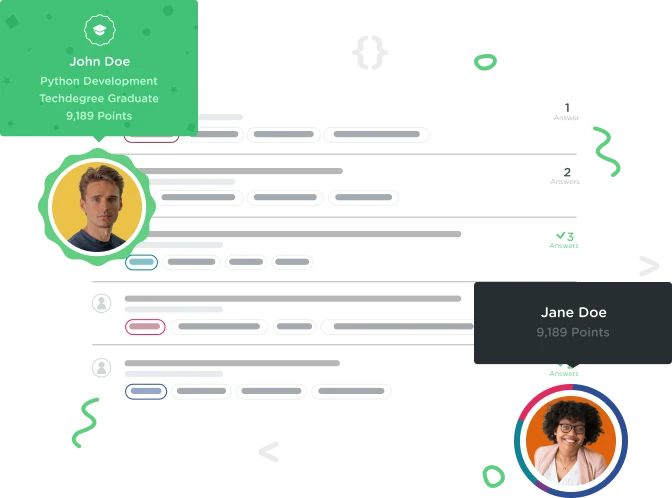
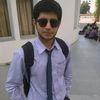
Neelesh Tewani
1,239 Pointschallenge 2 of 3 ?
// bad intializer and inputstreamreader error
package com.example.model;
import java.util.List;
import java.util.Set;
import java.util.HashSet;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Course {
private String mTitle;
private Set<String> mTags;
public Course(String title) {
mTitle = title;
// TODO: initialize the set mTags
mTags = new HashSet<String>();
}
public void addTag(String tag)throws IOException {
// TODO: add the tag
BufferedReader mReader = new BufferedReader(InputStreamReader(System.in));
tag= mReader.readLine();
for(tag: mTags){
try{
tag.add(mTags);
}catch(IOException ioe){
System.out.println("there is some problem in your input");
}
}
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
}
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
return false;
}
public String getTitle() {
return mTitle;
}
}
3 Answers
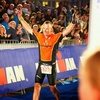
Steve Hunter
57,712 PointsHi there,
For addTag
you just use the add
method on mTags
- there's only one string to use, the parameter passed in. For the addTags
method, which takes a list as a parameter, you need to set up a loop to iterate through each tag
in the list, then use the first method, addTag
, to add each one individually:
public void addTag(String tag) {
mTags.add(tag);
}
public void addTags(List<String> tags) {
for (String tag : tags){
addTag(tag);
// same as: mTags.add(tag);
}
}
Make sense?
Steve.

Andrew Dummer
6,885 PointsThe input is going into tag
when you read the line, so that is what you want to add by substituting mTags.add(tag);
where you currently have written tag.add(mTags);

Mariette Grans
4,279 PointsI tend to make it more difficult than it really is. Thank you
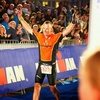
Steve Hunter
57,712 Points