Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial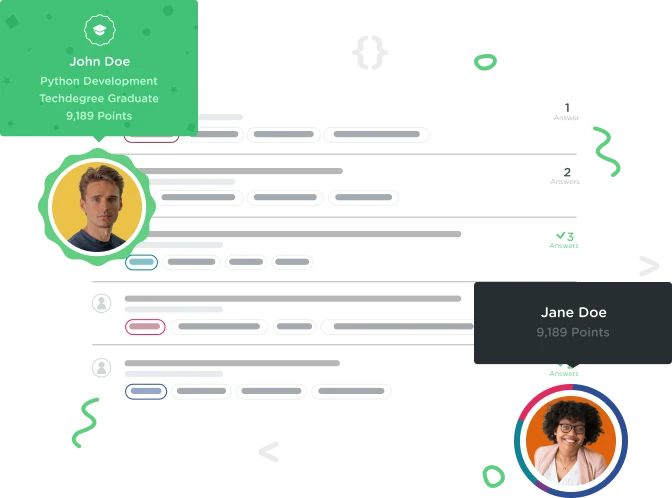

Nas Jones
7,849 PointsChallenge
I haven't coded in a while so i'm kind of confused. Just to be sure this objective is asking me to add a class called highlight to the immediate previous sibling of every <p> tag?. I don't know what's being asked and i don't know how to execute it because it's been a while
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
3 Answers

Blake Larson
13,014 PointsIf the button
in the list item is pushed they want you to add the highlight
class to the p
element in the same list item.
e.target.previousSibling.classList.add('highlight');

Blake Larson
13,014 PointsSo the p
element is inside the li
element with the button
. When you use an event listener like click
the function you call inside that listener can take a event object which has a target
which is the element you clicked on.
The previousSibling
property returns the previous node of the specified node, in the same tree level, which means if two elements are in a parent li
the second element can use this call to grab the reference of the first sibling. If there are 3 siblings the 3rd sibling can call the 2nd sibling and so on.
Each element in the DOM has a classList which makes it easy to add and remove classes from elements with JavaScript.
https://www.w3schools.com/jsref/prop_element_classlist.asp <-- for more info

Nas Jones
7,849 PointsI get what you were saying for the previousSibling and the classList, I still don't see how the <p> element has anything to do with anything. Why would they even mention it in the objective, because like i said i noticed in the code the <p> element wasn't used

Blake Larson
13,014 PointsNot exactly sure what you're asking. The li
element is the parent element to the p
and button
elements. Since the button
is after the p
in the HTML code, the p
element is the previous sibling of the button
in the parent li
. The challenge wants the p
element to get a class added to it for styling.
If you switched the p
and button
elements in the HTML it wouldn't work because the button
would be the first child and the previousSibling call wouldn't work. Would need to use nextSibling
to access the p
.
Hopefully that didn't make it even more confusing.
Could also look at it like this if you don't understand where the p
element is being used,
let previousParagraphElement = e.target.previousSibling; // Grabs the paragraph element
previousParagraphElement.classList.add('highlight');
Nas Jones
7,849 PointsNas Jones
7,849 PointsHey thanks for helping! this solved it, but just so I'm sure and you can correct me if I'm wrong cause i still don't kind of understand what's going on. Why is the "e.target" part necessary?, also the "classList.add" part is that a way of adding a class name to something?, and if we're targeting the button where does the <p> element come into all of this? Cause i noticed in the code above that you helped with the "<p>" element wasn't there