Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial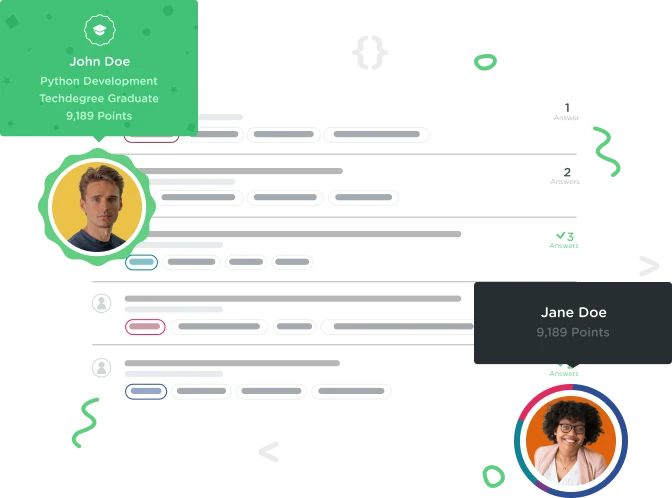

Avi Tsipshtein
7,461 PointsChallenge 4/4, Python collection. Is there another way to add items to list other then append() or extend()?
Hi Guys,
I ran into a weird problem. I need to create a list of all the values in the dictionary. When I use append() I get this error: "You returned 9 courses, you should have returned 18." When I use insert() I get: "You returned 25 courses, you should have returned 18." Is there another way to add add items into a list without needing to specify an index (since the dictionary has no indexes)?
here is my code:
my_dict = {
'Kenneth Love': ['Python Basics', 'Python Collections'],
'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Me Me': ['My Course'],
'hiroshe': ['My Course'],
}
def most_classes(my_dict):
max_count = 0
busy_teacher = ' '
for item in sorted(my_dict, key=lambda item:len(my_dict[item]), reverse=True):
if len(my_dict[item]) > max_count:
max_count = len(my_dict[item])
busy_teacher = item
return busy_teacher
most_classes(my_dict)
def num_teachers():
teach_count = 0
for key in my_dict:
teach_count += 1
return teach_count
num_teachers()
def stats():
stats_list = []
for key, value in my_dict.items():
temp_list = [key, len(value)]
stats_list.append(temp_list)
return stats_list
stats()
course_list = []
def courses(my_dict):
for key, value in my_dict.items():
course_list.extend(value)
return course_list
courses(my_dict)
thanks!
2 Answers
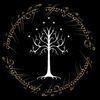
jacinator
11,936 PointsI got these working using list comprehension for the most part.
dictionary = {
'Kenneth Love': ['Python Basics', 'Python Collections'],
'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Me Me': ['My Course'],
'hiroshe': ['My Course'],
}
def most_classes(dictionary):
count = 0
teacher = None
for teacher in dictionary:
if len(dictionary[teacher]) > count:
count, teacher = dictionary[teacher], teacher
return teacher
most_classes(dictionary)
def num_teachers(dictionary):
return len(dictionary.keys())
num_teachers(dictionary)
def stats(dictionary):
return [[key, value] for key, value in dictionary.items()]
stats(dictionary)
def courses(dictionary):
return sum([len(courses) for courses in dictionary.values()])
courses(dictionary)
Not that I expect you to follow this exactly, but it may give you ideas to fix your bugs. If I had to guess where your bug is I'd say it's in putting course_list
outside of courses()
.
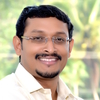
Aby Abraham
Courses Plus Student 12,531 Pointsdef most_classes(dict):
max_count = 0
str = ""
alist = dict.values()
for key, value in dict.items():
if len(value) > max_count:
max_count = len(value)
str = key
return str
def num_teachers(dict):
return len(dict.keys())
def stats(dict):
slist = []
for key, value in dict.items():
slist.append([key, len(value)])
return slist
def courses(dict):
clist = []
for value in dict.values():
clist.extend(value)
return clist
Avi Tsipshtein
7,461 PointsAvi Tsipshtein
7,461 PointsThanks jacinator! Your guess was correct but why? In other places it excepts the global list and doesn't create a bug. Also, it ran fine on my IDE as is. Can you explain what is the difference if I put the list outside the function or inside the function?