Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial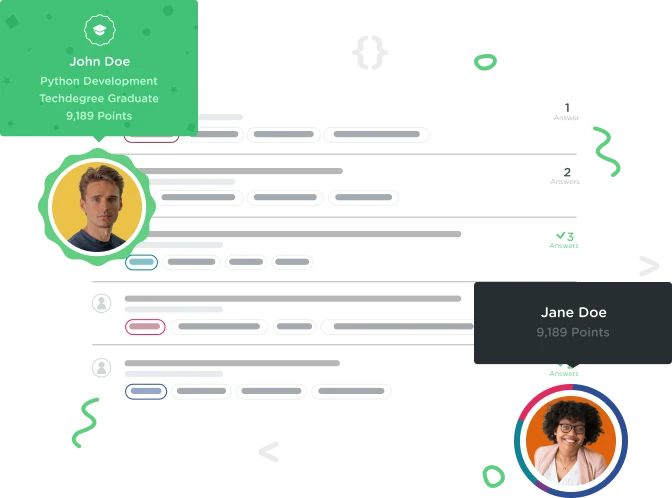
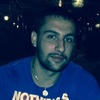
Bogdan Rancichi
4,464 PointsChallenge for Comparable interface error
When i create my code for resolving this challenge, it says that Task 1 is not being resolved, but in Task 1 it says that you have to always return 1, so now if a return -1 its an error, I don't understand whats the problem.
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
public int compareTo ( Object obj) {
BlogPost b = (BlogPost) obj;
if (b.equals(this)) {
return 0;
}
if ( b.mCreationDate.compareTo(this.mCreationDate) <= 0) {
return -1;
}
return 1;
}
}
5 Answers
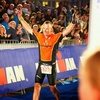
Steve Hunter
57,712 PointsFinito ...
public int compareTo(Object obj){
BlogPost bp = (BlogPost) obj;
if(this.equals(obj)){
return 0;
}
if(this.mCreationDate.before(bp.mCreationDate)){
return -1;
}
return 1;
}
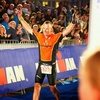
Steve Hunter
57,712 PointsI had the same problem when trying to help someone else with this challenge. The post for the is here - there may be something useful in there for you.
One thing, you can't call the compareTo
method inside its own definition.
I'll see if I can get through the challenge and add more help to this!
Steve.
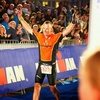
Steve Hunter
57,712 PointsRight - the following code passes the second part of the challenge without it reverting to failing task 1:
public int compareTo(Object obj){
BlogPost bp = (BlogPost) obj;
if(this.equals(obj)){
return 0;
}
return 1;
}
I don't think the new variable bp
is required - I'll try with it deleted.
I'll keep going!
Steve.
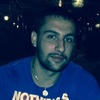
Bogdan Rancichi
4,464 PointsThank you, for your answer, but i still don't know why my solution was wrong.
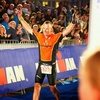
Steve Hunter
57,712 PointsI'll be honest; I have no idea what was going on in that one!
However, in your solution, you were using compareTo
within the definition of the compareTo
method:
public int compareTo ( Object obj) {
BlogPost b = (BlogPost) obj;
if (b.equals(this)) {
return 0;
}
if ( b.mCreationDate.compareTo(this.mCreationDate) <= 0) { // <-- compareTo within compareTo?
return -1;
}
return 1;
}
So, if you'd got the correct comparator to test the order of the two dates, the rest was fine. I used after
initially, but that failed, so I switched to before
.
Steve.
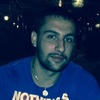
Bogdan Rancichi
4,464 PointsUsing compareTo in compareTo is not a issue because i am implementing the compareTo for the BlogPost and then i use post.mCreationDate.compareTo , and the second compareTo is from the Date object already implemented in Java.
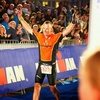
Steve Hunter
57,712 PointsAh right - I didn't realise compareTo
was already a method of the Date
class.
The whole challenge could use the output of that within a switch
statement. Switch the result of the compareTo
for the three cases:
- < 0 return -1
- 0 return 0
- >0 return 1
In your compareTo, did you get the greater/less than direction correct? I've been looking at this too long now, so can't get my head round the date differences.
Steve.
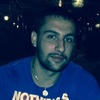
Bogdan Rancichi
4,464 PointsYes i did return -1 when the compareTo() < 0, but never mind it works with .before, thanks Steve.
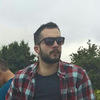
Rui Peixoto
22,820 Pointspublic int compareTo ( Object obj) {
if (equals(((BlogPost) obj)) {
return 0;
}
if ( mCreationDate.before(((BlogPost) obj).mCreationDate)) {
return -1;
}
return 1;
}
Mark Buckingham
5,574 PointsMark Buckingham
5,574 PointsI do not have a compiler at hand to check, but couldn't this be simplified by just returning the date.compareTo() result?
Bogdan Rancichi
4,464 PointsBogdan Rancichi
4,464 PointsYes it could be, but in some strange way it doesn't respect Task 1. I think this is an error from their validation.
Mark Buckingham
5,574 PointsMark Buckingham
5,574 PointsYes it seems the challenges want the answer written in a specific way, which is fair as trying to cover all the possible permutations would be quite a task.
Abirbhav Goswami
15,450 PointsAbirbhav Goswami
15,450 PointsBut I don't remember learning the 'this' keyword. Thanks though!