Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial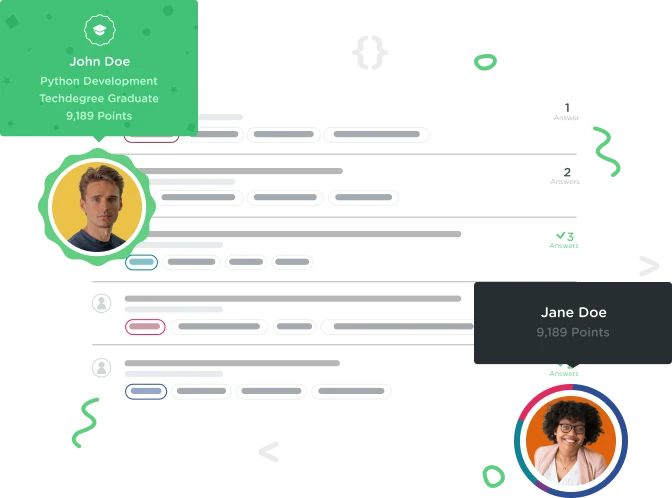

saro lin
Courses Plus Student 531 Pointschallenge on instance metods
I know there are some errors in my program. But I cant figure it out. Please help me:
class Game:
player = 1
def score(self,player):
self.player=self.player+1
return self.player
def __init__(self):
self.current_score = [0, 0]
[MARKDOWN ADDED BY MOD]
2 Answers
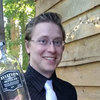
Evan Demaris
64,262 PointsHi saro,
It's a little difficult to tell what your goal is with that code.
Are you trying to say that there is more than one player in your game Class?
If not, why does your init include a list with two numbers?
If so, why would you only increment one player's score and not update anything to the current_score?

Iain Simmons
Treehouse Moderator 32,305 PointsSo, the problem is, you're actually using 3 different types of variables called player
.
First, you've got a local variable within the class Game
, which will be an attribute of the class itself. It should instead be initialised with a default value when an instance of the class is created (put it in the __init__
function).
Second, you've got a parameter that is expected to be passed as an argument to the score
function, which doesn't get used.
Third, you've got self.player
, which would be an attribute of an instance of that class. It will use the value of the class attribute, since it didn't get one set for the instance itself.
I'd suggest changing your code to something like the following, depending on what your intention was.
If you only want one player's score being recorded:
class Game:
def __init__(self):
self.player = 1
def score(self):
self.player = self.player + 1
return self.player
Used like this:
game = Game()
# game.player == 1
game.score()
# game.player == 2
game.score()
# game.player == 3
If you want to record the score of two competing players:
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self, player):
self.current_score[player] += 1
Used like this:
game = Game()
# game.current_score == [0, 0]
# increase first player's score (index 0)
game.score(0)
# game.current_score == [1, 0]
# increase first player's score again
game.score(0)
# game.current_score == [2, 0]
# increase second player's score (index 1)
game.score(1)
# game.current_score == [2, 1]