Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial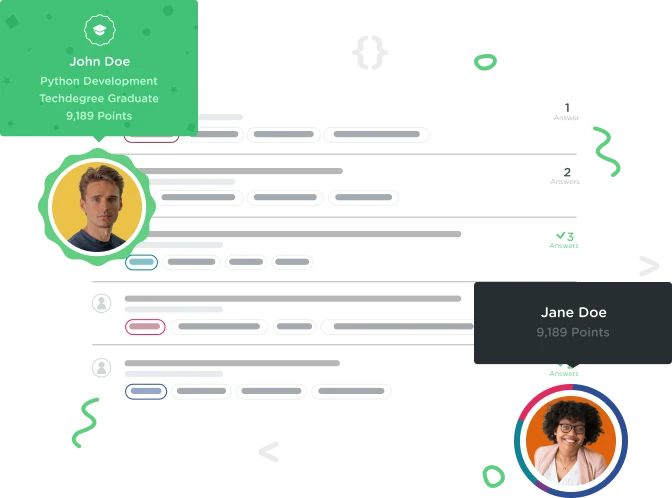
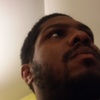
Alex Lowe
15,147 PointsChallenge Part 1
I'm getting these errors:
./com/example/BlogPost.java:3: error: class, interface, or enum expected package com.example; ^ JavaTester.java:39: error: cannot access BlogPost Field[] fields = BlogPost.class.getDeclaredFields(); ^ bad source file: ./com/example/BlogPost.java file does not contain class com.example.BlogPost Please remove or make sure it appears in the correct subdirectory of the sourcepath. 2 errors
What did I do wrong?
import java.util.Date;
package com.example;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mCategory;
private Date mCreationDate;
}
5 Answers

Craig Dennis
Treehouse TeacherPut the package declaration as the first line. Sorry 'bout that weird error. Will recreate and fix!

james white
78,399 PointsHi Alex,
Just to let you know, even if you put the package declaration first your code is still off.
Here's the link to the challenge:
http://teamtreehouse.com/library/java-data-structures/getting-there/class-review-2
The challenge question is:
Add declarations for private member fields for author, title, body, category, and creation date. The date should be of type java.util.Date (make sure you import it).
So there are 5 declarations needed:
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
}
I'm stuck on part two of the challenge:
Challenge Task 2 of 3
Now add a constructor that accepts author, title, body, category and creation date. In your constructor, initialize the private variables you created in Step 1 using the arguments passed in.
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategoy = category;
mCreationDate = creationDate;
}
}
It's giving me a syntax errors:
./com/example/BlogPost.java:13: error: class, interface, or enum expected public BlogPost(String author, String title, String body, String category, Date creationDate) { ^
./com/example/BlogPost.java:15: error: class, interface, or enum expected mTitle = title; ^
./com/example/BlogPost.java:16: error: class, interface, or enum expected mBody = body; ^
./com/example/BlogPost.java:17: error: class, interface, or enum expected mCategoy = category; ^
./com/example/BlogPost.java:18: error: class, interface, or enum expected mCreationDate = creationDate; ^
./com/example/BlogPost.java:19: error: class, interface, or enum expected } ^
6 errors
Also tried it like this:
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
}
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategoy = category;
mCreationDate = creationDate;
}

Yorick ter Heide
1,744 PointsPut the constructor inside of the BlogPost Class.
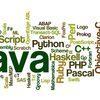
Bilal Ron
2,323 PointsFor me this worked
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
}

Jordan Scott
16,680 PointsMake sure to put you Constructor inside of the BlogPost Class
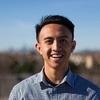
Gendarme Docena
1,509 PointsHi, for the first task, how come "private Date creationDate;" doesn't need a string? Is it because you are importing?

Chris Smith
3,719 PointsHi Gendarme, Probably a bit late, but the 'Date' is the type declaration.
Alex Lowe
15,147 PointsAlex Lowe
15,147 PointsThanks Craig.
Akash yadav
10,046 PointsAkash yadav
10,046 PointsHey Craig, that weird error still exists! Please look forward to fix it.
Ethan Goodwin
6,537 PointsEthan Goodwin
6,537 PointsThat error is still existing.
Neiko Frye
3,840 PointsNeiko Frye
3,840 PointsCraig Dennis Please allow us to use "This" keyword rather than mVariableName.