Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial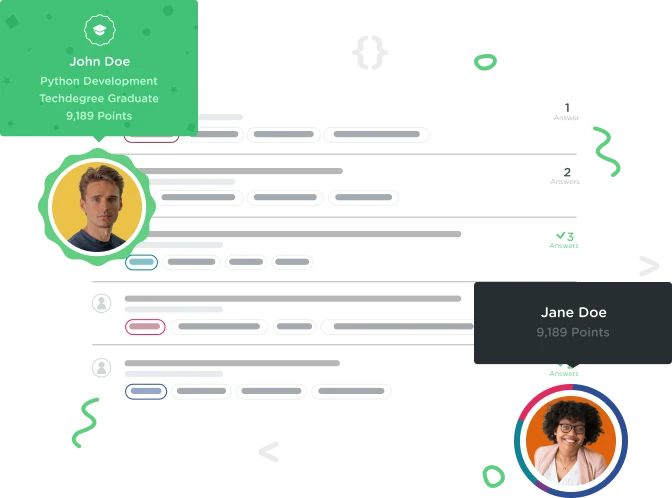

Adam Howell
601 PointsChallenge saying I am wrong but my code works on my own.
I am working on the coding challenge for Methods 2 and the second task will not let me pass even though my code gives me the correct output when I try it within my on example code outside of treehouse.
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying2.sort(function (a,b) {
return a.length > b.length;
});

Adam Howell
601 Pointsadded my code
4 Answers
Andrew McCormick
17,730 PointsI believe it should be return a.length - b.length;
. I'm not a JS expert so I don't know if the ">" should work or not, but I know "-" does.
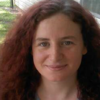
Cherie Burgett
8,711 Pointsthe ">" would work in an if statement. ie if (a.length > b.length) return 1;
if (a.lenth < b.length) return -1;
if (a.length == b.length) return 0;
please correct me if i'm wrong I'm still new too. edited typo

Stone Preston
42,016 Points>
would work in a return statement as well, the boolean statement is evaluated first and the value is returned
return a.length > b.length;
would return either true or false depending on the value of a.length and b.length

Adam Howell
601 PointsSo I need to just change my ">" to a "-" and this will work within the challenge?

Jason Anello
Courses Plus Student 94,610 Points@Stone,
Adam claims that this works outside of the code challenge and you're claiming that it works too, but how? The function is returning a boolean value but it needs to return a positive, negative, or zero value so that the sort method can determine whether 'a' should come before b
or not. Cherie's long form representation of the answer shows this explicitly. Not sure how returning a boolean will duplicate that functionality.
Does it have to do with the numerical representations of true and false? Will the sort method convert the true/false values to numbers?
@Cherie, that's correct. That's the general form of the compare function. In this case, a.length - b.length
is simply a shorter way of achieving the same functionality. You actually don't need the third if
. You can simply return 0
at the end of the function because if you've made it that far, they have to be equal.

Stone Preston
42,016 PointsI did not say that its the correct implementation for this problem. I was just replying to cherie that boolean operators like >
can be used in more than just if statements., (but i misinterpreted her comment somewhat )
I said "> would work in a return statement" not "> would work in this return statement"
sorry if I confused anybody haha

Jason Anello
Courses Plus Student 94,610 PointsOk, thanks for clarifying. Sorry, I misinterpreted your comment too.
Well, at least Adam has 2 correct answers now. That's the important thing.

Adam Howell
601 PointsSo I need to just change my ">" to a "-" and this will work within the challenge?

Jason Anello
Courses Plus Student 94,610 PointsYes, that's correct.
I am curious as to how your original code worked outside the challenge. It actually sorted the array correctly without any errors?

Adam Howell
601 Pointsyes, worked perfectly fine.
Andrew McCormick
17,730 Pointswho knew (well, looks like Adam did :) ) This is what it looks like in Chrome console:

Adam Howell
601 PointsAndrew Chalkley Thats what I got in Chrome console as well.
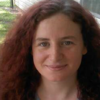
Cherie Burgett
8,711 PointsHow Fun! Who knew coding to be this exciting thanks guys you're the best! I think we've all learned something here. Thank you Jason for your explaination.

Jason Anello
Courses Plus Student 94,610 PointsReturning a boolean expression from the compare function isn't always going to work. This particular example works in chrome.
Since this is working in chrome with returning a boolean from the compare function then the sort method must be converting true and false to numbers, 1 and 0 respectively. This means you have lost the ability to return a negative value which is necessary to let the sort method know that a
must come before b
. Here, a
and b
are treated as equal even if a
should come before b
This might be fine if the sort method is stable.
See the card diagram on the right in the "Stability" section: https://en.wikipedia.org/wiki/Sorting_algorithm#Stability to see what happens in a stable sort and what might happen in an unstable sort. The 5's could get reversed from their original order.
The ECMAscript specification states that the sort method isn't necessarily stable. It also doesn't specify any particular sorting algorithm to be used.
source: http://ecma262-5.com/ELS5_HTML.htm#Section_15.4.4.11
So it's really up to individual implementers on what algorithms are going to be used and whether those algorithms are stable/unstable.
I decided to do a little research to see if there were any unstable implementations. Based on what others have said, IE9, android 2.3, and some versions of Opera had unstable sorts. I tried IE10 and it seems to be unstable as well or doesn't like receiving a boolean.
Chrome seems to implement a stable sorting algorithm with 10 elements or less but switches to an unstable sort with more than 10 elements. As luck would have it, the array in this challenge is exactly 10 elements. Add 1 more and chrome no longer sorts it correctly when returning a boolean from the compare function .
//Chrome console
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back", "11"];
saying2.sort(function (a,b) {
return a.length > b.length;
});
["over", "11", "fox", "the", "The", "lazy", "back", "brown", "quick", "dog's", "jumped"]
It does sort this correctly with a proper compare function although it doesn't necessarily preserve the original order of equal items due to the unstable sort.
This also means that even with a proper implementation of the compare function, firefox and chrome won't necessarily produce the exact same output.
// Chrome console
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back", "11"];
saying2.sort(function (a,b) {
return a.length - b.length;
});
["11", "The", "the", "fox", "lazy", "back", "over", "quick", "brown", "dog's", "jumped"]
// Firebug console in firefox
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back", "11"];
saying2.sort(function (a,b) {
return a.length - b.length;
});
["11", "The", "fox", "the", "over", "lazy", "back", "quick", "brown", "dog's", "jumped"]
They're both technically sorted correctly but Chrome doesn't preserve the original order of equal items.
So i think you're taking a risk if you return a boolean from the compare function.
Andrew McCormick
17,730 PointsAndrew McCormick
17,730 PointsAdam, Please always share your code you are using in the challenge so we can see what you are working with. thanks How to post code in forum