Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial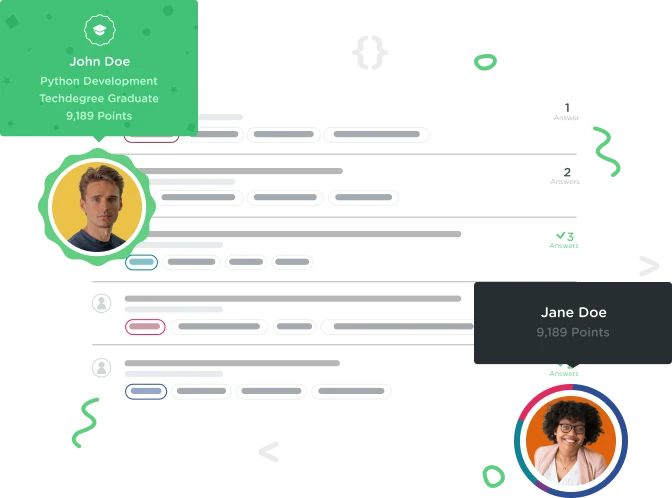

boris said
3,607 PointsChallenge saying that I need to make a custom initializer but I already have.
Also, there are no errors in X-Code. I appreciate your help greatly and thank you in advanced.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
3 Answers
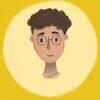
Ben Bastow
24,657 PointsHi!
Your code is syntaxlly correct! but its not correct for the challenge. You dont need to init the description so your code should look like this
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
The reason you don't init the description is because it says to only init the first 4 properties. (I hope I have made sense, if i haven't please tell me and il try and explain it in a different way!)
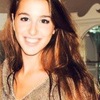
Sonia Feldman
3,873 PointsHi Boris,
In your code, you are currently setting the self values to constant Doubles. Those numbers are given in the challenge as an example. The struct should be able to return any set of Double values, not just 86.0, 191.0 etc.
To make the code flexible, you need to assign the stored color properties (self.red, self. green etc) to the color values passed in by the initializer method (red, green etc).
The proper code looks like this.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
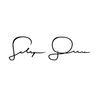
Filip Diarra
6,647 PointsQuick question: If we don't initialize the description, how can this work? I thought that if you initialize manually, you must initialize all of the constants.
Does that make sense? Thanks!
boris said
3,607 Pointsboris said
3,607 PointsI understand, thank you for helping me.