Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial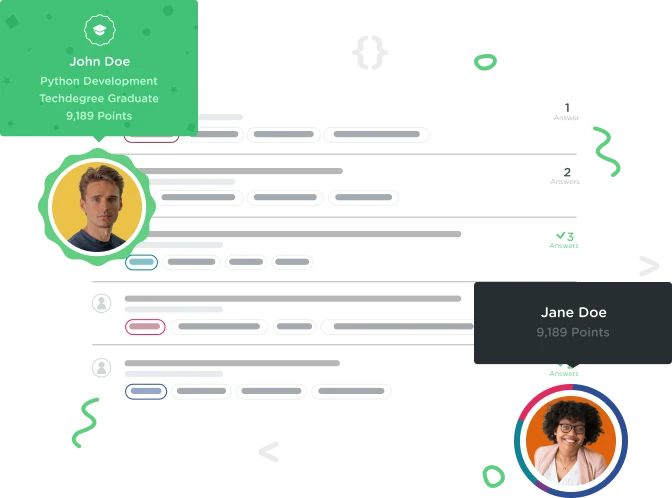

prateekparekh
12,895 PointsChallenge score_yatzy
if there is a yatzy (5 dices with the same number), then length of one of these lists would be 5. What am I missing here?
def score_yatzy(self, hand):
if 5 in {len(hand.ones), len(hand.twos), len(hand.threes), len(hand.fours), len(hand.fives), len(hand.sixes)}
return 50
else:
return 0
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def score_ones(self, hand):
return sum(hand.ones)
def score_ones(self, hand):
return sum(hand.ones)
def score_ones(self, hand):
return sum(hand.ones)
def score_ones(self, hand):
return sum(hand.ones)
def score_sixes(self, hand):
return sum(hand.sixes)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
return sum(hand.ones) + sum(hand.twos) + sum(hand.threes) + sum(hand.fours) + sum(hand.fives) + sum(hand.sixes)
def score_yatzy(self, hand):
if 5 in {len(hand.ones), len(hand.twos), len(hand.threes), len(hand.fours), len(hand.fives), len(hand.sixes)}
return 50
else:
return 0
4 Answers

billy mercier
6,259 PointsHope this helps, make sure you understand!
def score_yatzy(self, hand):
dicelist = [] #a list to append same value dice
for dice in hand: #looping through to check all dice
dicelist.append(dice)
if dices == hand[0]: #you need to compare all dice
dicelist.append(dice) #only append in list if they are the same
if len(dicelist) == 5: #if that list gets 5 dice of same value = yatzy
return 50
else: #if any of those dice didn't have the same value as hand[0] != yatzy
return 0

cb123
Courses Plus Student 9,858 PointsAnother option is to review what the _score_set method was doing and borrow that approach. Within that function there is hand._sets.items() which has captured a dictionary of the dice rolls and their associated counts and which we can loop through to find a count of 5.
def score_yatzy(self, hand):
for worth, count in hand._sets.items():
if count == 5:
return 50
return 0
Hopefully this helps in seeing the solution in a different way.

Oluwatominiyin Adebanjo
3,223 PointsAnother solution
def score_yatzy(self, hand):
for die in hand:
if hand.count(die) >= 5:
return 50
return 0

Adam Kielar
Python Web Development Techdegree Graduate 13,818 PointsHere is my solution: ''' def score_yatzy(self, hand): if hand[1:] == hand[:-1]: return 50 else: return 0 '''
prateekparekh
12,895 Pointsprateekparekh
12,895 PointsWhy are you appending twice? dicelist.append(dice)
Is that a typo?