Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial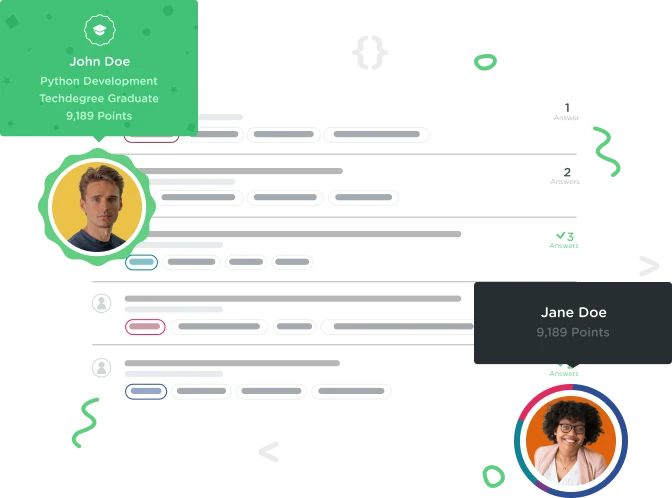
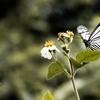
Justin Kao
5,724 PointsChallenge Solution: Only ONE more Listener
Below is my solution.
I add 2 custom functions to re-render the list, and remove first / last element corresponding button.
In listener section, I add a listener on parent div.list
to capture the event from child ul
.
Because event bubbling, the div
listener handler will always re-render after children event handler triggered.
However, I think there is a performance issue here, because I need to render entire list each time. Still working on how to only partially changing some of the attributes.
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const listUl = listDiv.querySelector('ul');
const ulChildren = listUl.children
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
/* main function*/
reRenderList()
/* functions*/
function reRenderList () {
console.log(ulChildren[0].innerText.split('\n')[0])
for (let i = 0; i < ulChildren.length; i++) {
ulChildren[i].innerHTML = `
${ulChildren[i].innerText.split('\n')[0]}
<button class="up">Up</button>
<button class="down">down</button>
<button class="remove">Remove</button>`
}
const firstLiUp = listUl.firstElementChild.children[0]
const lastLiDown = listUl.lastElementChild.children[1]
// firstLi.style.backgroundColor = 'coral'
// lastLi.style.backgroundColor = 'aliceBlue'
cleanBtn(firstLiUp, lastLiDown)
}
function cleanBtn (firstEle, lastEle) {
firstEle.remove()
lastEle.remove()
}
/* listeners*/
// my code
listDiv.addEventListener('click', (e) => {
if (e.target.tagName === 'BUTTON'){
reRenderList()
}
})
// code in course
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
}
if (event.target.className == 'down') {
let li = event.target.parentNode
let nextLi = li.nextElementSibling
let ul = li.parentNode
if (nextLi){
ul.insertBefore(nextLi, li)
}
}
}
});
toggleList.addEventListener('click', () => {
if (listDiv.style.display == 'none') {
toggleList.textContent = 'Hide list';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show list';
listDiv.style.display = 'none';
}
});
descriptionButton.addEventListener('click', () => {
descriptionP.innerHTML = descriptionInput.value + ':';
descriptionInput.value = '';
});
addItemButton.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.innerHTML = `${addItemInput.value}
<button class="up">Up</button>
<button class="down">down</button>
<button class="remove">Remove</button>
`;
ul.appendChild(li);
addItemInput.value = '';
});