Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial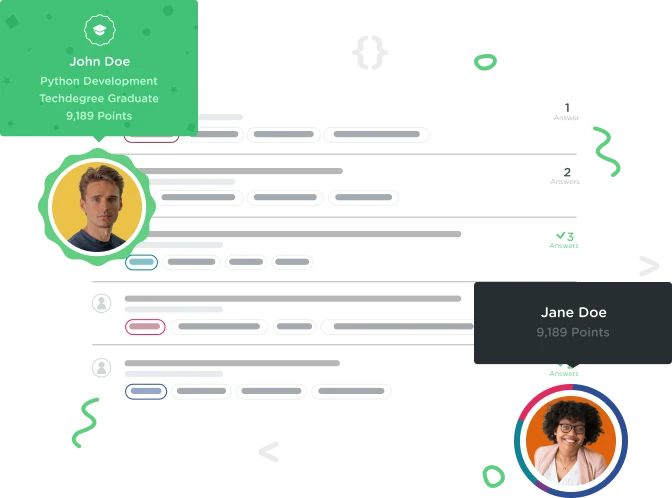

João Albuquerque
1,642 PointsChallenge task 1
I got this error:
./TeacherAssistant.java:8: error: illegal start of expression if (fieldName.charAt(0) != 'm') && (Character.isUpperCase(fieldName.charAt(1)))) { ^ ./TeacherAssistant.java:8: error: ';' expected if (fieldName.charAt(0) != 'm') && (Character.isUpperCase(fieldName.charAt(1)))) { ^ 2 errors
what is wrong ?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm') && (Character.isUpperCase(fieldName.charAt(1)))) {
throw new IllegalArgumentException("Error!");
}
return fieldName;
}
}
4 Answers
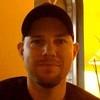
Jeremy Hill
29,567 PointsThis is what I did:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm' || !Character.isUpperCase(fieldName.charAt(1))){
throw new IllegalArgumentException("Not a valid field name!");
}
return fieldName;
}
}

João Albuquerque
1,642 PointsAh yes , I thought I had to separate each expression with parentheses and I forgot that in the beginning. But one more question ... I should not use && as a member variable must start with 'm' AND the second letter must be uppercase.
I'm sorry for any grammar mistake, I 'm from Brazil :)
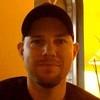
Jeremy Hill
29,567 PointsWhen you have an if statement with two conditions you will have either '&&' (and) or 'll' (or); when you use && this means that BOTH conditions HAVE to be met, and when you use 'll' only one of the two conditions need to be met, here is an example:
If(age <= 50 && wrinkles < 5) System.out.println("Not that old");
If(age > 50 ll wrinkles >=5) System.out.println("Kind of old")
I hope this helps.
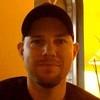
Jeremy Hill
29,567 PointsAlso, the field name being referred to is a member variable, also known as a instance variable. The way treehouse teaches it is when you are declaring a member variable you use a lowercase 'm' followed by the name of your variable with the first letter of the name being capitalize; so if you were to declare a member variable for the word "size" it would look like this: mSize
The challenge wants you to make sure that whatever is passed in gets checked using the if statement to see if the first letter is a lowercase 'm' and the next letter is capitalized; if it isn't in that format an exception will be thrown.

João Albuquerque
1,642 PointsWow , now I understand it all . Thank you very much!
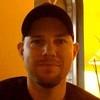
Jeremy Hill
29,567 PointsYou're welcome :)
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsOne thing I noticed is your parenthesis- for your if statement you need an opening and a closing for everything inside; the closing one in your code was after the 'm' so everything after that was confusing the compiler.
Also I changed the '&&' to '||' that way either condition will throw the error instead of having to have a capital 'm' and an upper case letter next to it.
Also, I noticed that you didn't have a '!' in your second condition in your if statement so the program would have been looking to throw an exception had the second character been upper cased.