Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial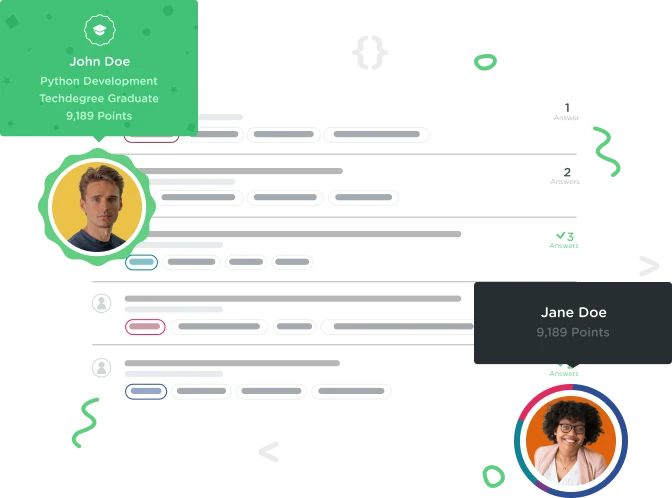

Jed Piezas
1,411 PointsChallenge Task 1 help
Hi all,
I'm trying to solve Challenge Task 1 but I'm not sure how I should begin. There are a few things that elude me at the moment:
What argument would my functions take? I was thinking it could be []. Do I also need to use the splat operator like I did in my code?
Where does the "for" loop go?
Thanks, I appreciate any help!
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
def add_list(*args):
return sum(args)
def summarize(*args):
print("The sum of " + args + "is " + sum(args))
3 Answers
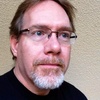
Chris Freeman
Treehouse Moderator 68,423 Points- What argument would my functions take? I was thinking it could be []. Do I also need to use the splat operator like I did in my code?
Task 1 asks: Make a function named add_list that takes a list. "Takes a list" is coder-speak for a function that has one parameter, and you can expect that parameter to be a list. You can choose any name for this parameter in the function definition. It is helpful to choose something that has meaning, such as, input_list
or numlist
. Avoid using list
as a parameter name since list()
is a built-in function name. For example:
def add_list(numlist):
pass
There isn't a need for the "splat" or argument unpacking. This is used when a function can be called by a varying number of arguments.
- Where does the "for" loop go?
Since the task is to add up the elements in a list, the for
loop is inside the add_list()
function to iterate over the items in the list, adding each one to a current sum. In your code, you took advantage of the built-in function sum()
to directly add all the list items. For those that haven't found that function, a for
loop could be used:
def add_list(numlist):
total = 0
# add each item to sum
for item in numlist:
total = total + item
# return result
return total

Dan Johnson
40,532 PointsYour argument will be a list, so you won't want to pack anything.
The for loop was expected to be in add_list. The challenge isn't expecting the use of the built-in sum (Though it still passes).
For the summarize function I'd recommend using the format method. Otherwise you'll need to do explicit string conversions.

Jed Piezas
1,411 PointsThank you both for your answers! They were super helpful