Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial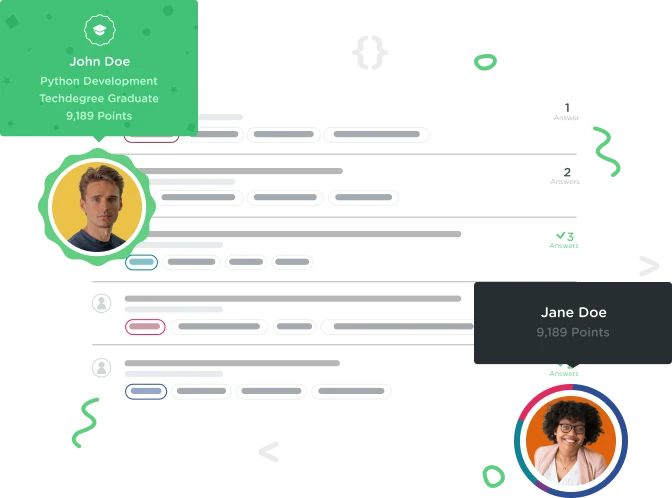
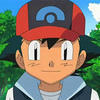
ASHOK Gudivada
11,701 PointsChallenge task 1 of 1 in word_count.py
String = "I do not like it Sam I Am"
>>> string_lower = String.lower()
>>> string_dict = {}
>>> for word in string_lower.split(" "):
... if word not in string_dict:
... string_dict[word] = string_lower.count(word+" ")
...
>>> string_dict
{'do': 1, 'like': 1, 'sam': 1, 'i': 2, 'am': 1, 'it': 1, 'not': 1}
>>>
it work but not passing, can someone help me plz
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string_lower = string.lower()
string_dict = {}
for word in string_lower.split(" "):
if word not in string_dict:
string_dict[word] = string_lower.count(word+" ")
return string_dict
6 Answers
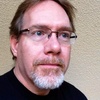
Chris Freeman
Treehouse Moderator 68,423 PointsThe task will not pass splitting on SPACE. It needs to split on Whitespace. Use split()
without arguments for this.
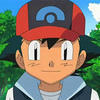
ASHOK Gudivada
11,701 Points>>> string_dict = {}
>>>
>>> my_str = "I see too many i's in this string"
>>> string_lower = my_str.lower()
>>> for word in string_lower.split(" "):
... if word not in string_dict:
... string_dict[word] = string_lower.count(word+" ")
...
>>> string_dict
{"i's": 1, 'string': 0, 'i': 1, 'many': 1, 'this': 1, 'see': 1, 'too': 1, 'in': 1}
please check this sir, it giving me right answer.
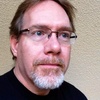
Chris Freeman
Treehouse Moderator 68,423 PointsIn your test string
has a count of zero. That's not correct. Using a SPACE is not sufficient for redundant suffixes and will miss the last word of the string if there is not a trailing space.
Try this to see the error: "The cat sat at the mat":
>>> word_count("The cat sat at the mat")
{'cat': 1, 'sat': 1, 'mat': 1, 'the': 2, 'at': 4}
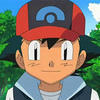
ASHOK Gudivada
11,701 PointsBut can I use count in some other way to complete the Challenge sir?
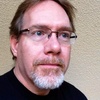
Chris Freeman
Treehouse Moderator 68,423 PointsUsing str.count()
will not work due to the false positives when a word is a subset of another word.
You are not far from the answer. Currently, you are:
- check if word has not been count yet
- scan string with
count
- store count in dict with
word
as key
What about trying:
- check if
word
not instring_dict.keys()
(not been counted) - if yes, then simply increment the count in the dict:
string_dict[word] += 1
- if no, then add the new word to the dict with an initial count of 1:
string_dict[word] = 1
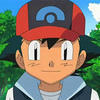
ASHOK Gudivada
11,701 PointsThank you *Chris Freeman*

Sameera Sy
2,349 PointsI have done the same thing as what Chris Freeman is suggesting, still no luck. Code runs in ideone with expected output but doesn't clear here! Not sure what this issue is about!
Ideone link : http://ideone.com/I6PpmI
def word_count(mystr):
myl = mystr.lower().split()
dic = {}
for value in myl:
if dic.has_key(value):
dic[value]+=1
else:
dic[value]=1
return dic
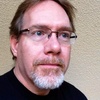
Chris Freeman
Treehouse Moderator 68,423 PointsThe dict.has_key()
was removed in Python 3. Use in
instead.
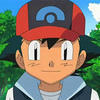
ASHOK Gudivada
11,701 Pointsdef word_count(mystr):
myl = mystr.lower().split()
dic = {}
for value in myl:
if value in dic:
dic[value]+=1
else:
dic[value]=1
return dic
my solution to the challenge
ASHOK Gudivada
11,701 PointsASHOK Gudivada
11,701 PointsBummer! Hmm, didn't get the expected output. Be sure you're lowercasing the string and splitting on all whitespace!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsTesting your code in the REPL reveals the issue:
Using
count()
is not specific enough. Try assigningstring_lower.split()
to a variable, then see ifword
isin
this list.To see the difference between
.split()
and.split("")
check the split docs