Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial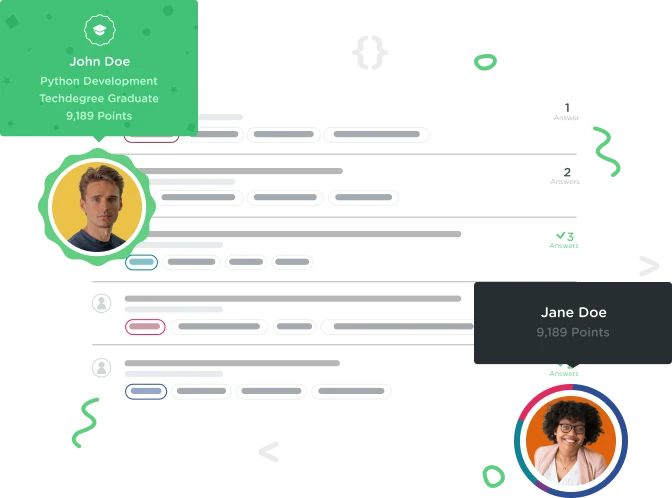
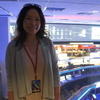
Yu-Ting (Scarlett) Liu
903 Pointschallenge task 1 of 1 in word_count.py
def word_count(a_string): string_dict = {} for word in a_string.split(): if word in string_dict: string_dict[word] += 1 else: string_dict[word] = 1 return string_dict
Something's wrong with my code??? I can't figure it out! Help!!
def word_count(a_string):
string_dict = {}
for word in a_string.split():
if word in string_dict:
string_dict[word] += 1
else:
string_dict[word] = 1
return string_dict
a_string = "I am what i am because of God"
a_string = a_string.lower()
print(word_count(a_string))
5 Answers

James Shi
8,942 PointsConvert the string to lowercase inside the function.
def word_count(a_string):
a_string = a_string.lower() # Add this line
string_dict = {}
for word in a_string.split():
if word in string_dict:
string_dict[word] += 1
else:
string_dict[word] = 1
return string_dict
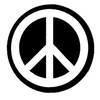
john larson
16,594 PointsHi James, how does that impact the outcome of the code? Or, why does that impact the outcome? Because, that clearly made this code pass the challenge. Wait, I get it... if the string is lowered outside the function, when Treehouse tests the code, whats outside the function won't be included. Good catch my friend.
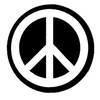
john larson
16,594 PointsHi Scarlett, I ran your code in my own editor and it looks like it works, but as you say it does not pass the challenge. I'm trying to remember, there was some nuance to this that made a lot of people not pass. I don't remember what it was.
# this is probably the most interesting solution I found
#{word: list_of_words.count(word) for word in list_of_words} <-- the parenthesis make it a dict
# word: list_of_words.count(word) <-- this sets up the dict.
# word is the key, list_of_words.count(word) is the value.
# .count is a built in function. It counts the occurrences of an item
# for word in list_of_words <-- just a for loop that iterates through and performs what was set up
def word_count(string):
list_of_words = string.lower().split()
string_dictionary = {word: list_of_words.count(word) for word in list_of_words}
return string_dictionary
string = "I am what I am because of God"

ds1
7,627 PointsHere's another way you could write it... see if this yields a different result:
a_string = "I am what i am because of God" a_string = a_string.lower()
def word_count(a_string): string_dict = {} for word in a_string.split(): if word in string_dict: string_dict[word] += 1 else: string_dict[word] = 1 print(string_dict)
word_count(a_string)

ds1
7,627 Pointsa_string = "I am what i am because of God"
a_string = a_string.lower()
def word_count(a_string):
string_dict = {}
for word in a_string.split():
if word in string_dict:
string_dict[word] += 1
else:
string_dict[word] = 1
print(string_dict)
word_count(a_string)
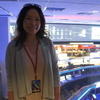
Yu-Ting (Scarlett) Liu
903 PointsI passed!!! Thank you guys for helping me solve the question! I guess I still have to practice more for these functions. :P
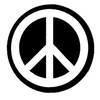
john larson
16,594 PointsThe challenges can be pretty tricky, and sometimes it seems that the challenge includes stuff not clearly defined, but we are expected to research it and find out.
john larson
16,594 Pointsjohn larson
16,594 PointsHi Scarlet, James submission below cleared it up for me. Anything outside the function will not be included when Treehouse tests the code.