Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial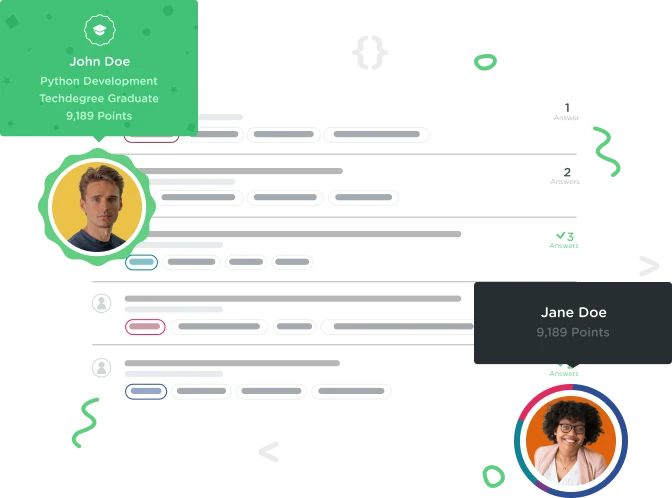

Anik Devaughn
7,751 PointsChallenge Task 1 of 1 phoneNumbers is array of 10 digit phone numbers, where the first three digits, in parentheses, ar
Hi, I have tried in many ways to solve this Challenge but no luck so far. And now I am Frustrated and need some help... Please can anyone tell me what mistake I am making here. Thanks in Advance !!! My Code: const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"]; let numberOf503;
// numberOf503 should be: 3 // Write your code below numberOf503 = phoneNumbers.reduce((count, name) => { if(name[0] === "503") { return count + 1; } return count; }, 0 );
//console.log(numberOf503);
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// numberOf503 should be: 3
// Write your code below
numberOf503 = phoneNumbers.reduce((count, name) => {
if(name[0] === "503") {
return count + 1;
}
return count;
}, 0 );
//console.log(numberOf503);
9 Answers

Valeshan Naidoo
27,008 PointsSo in this instance, you need think about whether name[0] is really going to give you "503", since the strings start with "(", and name[0] will search for the first character only. The better condition would be to use the startsWith method, with this, you can check for the beginning of any string and the value can be however long.
numberOf503 = phoneNumbers.reduce((numbers, number)=> {
if (number.startsWith('(503)')) {
return numbers+1} return numbers}, 0);
I could just even use startsWith('(5') if I wanted to.
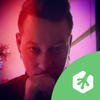
Athena Ozanich
Front End Web Development Techdegree Graduate 29,847 PointsFor that matter one could also use a conditional ternary to complete this task!
numberOf503 = phoneNumbers.reduce((numOfMatches, phnNum) => {
return ( phnNum.startsWith("(503)")?numOfMatches+1:numOfMatches)
}, 0);
NOTE: I used the .startsWith() method to check that the string begins with the desired search, I also included the parenthesis for the area code for good measure to help, ensure to sneaky little bugs get in >,<
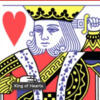
Bartlomiej Zabielski
Courses Plus Student 10,363 PointsnumberOf503 = phoneNumbers.reduce((acc, cur) => {
if (cur.substring(1, 4) === "503") {
return acc + 1;
} else {
return acc;
}
}, 0);
console.log(numberOf503);
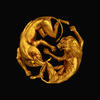
jonathanj
UX Design Techdegree Student 9,378 PointsThis is correct in that I used reduce to cycle through entire array. Having used an array method --> REDUCE <--- and having yielded the correct result, which in this case is 3 the tester should recognize my answer as correct should it not?
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// numberOf503 should be: 3
// Write your code below
phoneNumbers.reduce((count,areaCode)=>{
if(areaCode.includes('(503) ')){
count+=1
}numberOf503 = count;
return numberOf503;
},0);
Thanks in advance for any assistance/clarity members of the community provide. Blessings, Jonathan
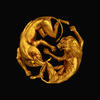
jonathanj
UX Design Techdegree Student 9,378 PointsI ended up going with this solution to satisfy the platform's tester...
numberOf503 = phoneNumbers.reduce((count,areaCode)=>{
if(areaCode.includes('(503) ')){
count+=1;
}
return count;
},0);```
...but I would still like to discuss what I initially crafted just above this post as well if possible.
Thanks much everyone.
Blessings, Jonathan

Ivo Culic
389 PointsOr one liner:
numberOf503 = phoneNumbers.reduce((acc, number) => (number.substring(1, 4) === "503") ? acc + 1 : acc, 0);
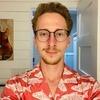
David Shulkin
Front End Web Development Techdegree Student 10,254 PointsI like this method! Thanks for sharing!

Anik Devaughn
7,751 PointsThanks alot ! It works

joakim edwardh
1,720 PointsI understand everything exept the 0 at the end. When i did the same code without the last 0 only the whole first number ("(503) 123-4567") was returned. Can someone please explain how the last 0 works?

Valeshan Naidoo
27,008 PointsThe last 0 is the base number in which the reduce function is able to accumulate from. For example, if we were totaling the cost of a list of items, the base can be set to 0 and then it adds from there. We could also have the total to whatever number we like. If the base number is omitted, the reduce function then starts at the value of the first item. In the case of the telephone numbers, we are calculating a total of the numbers beginning with a particular prefix, so we would like the base to be 0.
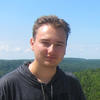
Sebastian Nadeau
Front End Web Development Techdegree Student 26,545 PointsThis is what I put as my answer. Why do we include + 1 in the if statement and 0 at the end of the function? Just to clarify, is the if statement after the arrow function part of a callback function? I would really appreciate someone pointing out the different parts of this code, as it gets a little confusing.
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// numberOf503 should be: 3
// Write your code below
numberOf503 = phoneNumbers.reduce((numbers, number) => {
if (number.startsWith('(503)')) {
return numbers + 1;
} return numbers;
},
0);
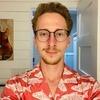
David Shulkin
Front End Web Development Techdegree Student 10,254 PointsHi all! You can solve this using the slice() method
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"]
let numberOf503
numberOf503 = phoneNumbers.reduce((count, number) => {
if (number.slice(0, 5) === "(503)" ) {
return ++count
}
return count
},
0)
Valeshan Naidoo
27,008 PointsValeshan Naidoo
27,008 PointsYou could also use your method, but with a little modification
Marcell Ciszek
7,255 PointsMarcell Ciszek
7,255 PointsThanks @Valeshan