Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial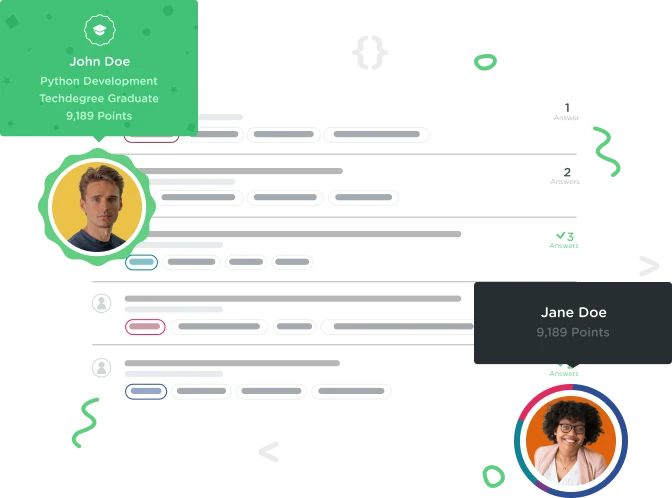
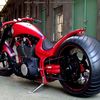
remslysambo
8,216 PointsChallenge Task 1 of 1 Write a function named members that takes two arguments, a dictionary and a list of keys.
Challenge Task 1 of 1
Write a function named members that takes two arguments, a dictionary and a list of keys. Return a count of how many of the items in the list are also keys in the dictionary.
Hi, i just fixed this code to pass the challenge, and i think that must be right way to do it. can somebody help please?
my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3} my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
def members(my_dict, my_list): count = 1 for fruit in my_dict: for fruits in my_list: if fruit == fruits: count += 1
return count
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
3 Answers
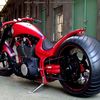
remslysambo
8,216 PointsThank you for your help :)
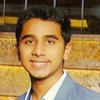
Anish Walawalkar
8,534 PointsHey remslysambo, here is an easier way to implement it:
def members(d, l):
count = 0
for item in l:
if d.get(item, None):
count = count + 1
return count
Steps:
- loop through every item in the list l
- the get function of the python dictionary d takes 2 arguments, the first being the key and second being a default value to return if a value for that key is not found
- for example my_lsit contains "strawberries" but my_dict does not contain the key "strawberries" hence when you call d.get("strawberries", None), it will return None and count will not get incremented
Hope that helps. :)

Ary de Oliveira
28,298 PointsFile testy25.py
def members(d, 1): count = 0
for item in 1: if d.get(item, None): count = count + 1 return count
treehouse:~/workspace$ python testy25.py
File "testy25.py", line 1
def members(d, 1):
^

Ary de Oliveira
28,298 PointsFile testy25.py
def members(d, 1): count = 0
for item in 1: if d.get(item, None): count = count + 1 return count
treehouse:~/workspace$ python testy25.py
File "testy25.py", line 1
def members(d, 1):
^

Lisa Burbon
1,030 Pointsdef members(my_dict, my_list):
count = 0
for item in my_dict:
if item in my_list:
count += 1
return (count)