Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial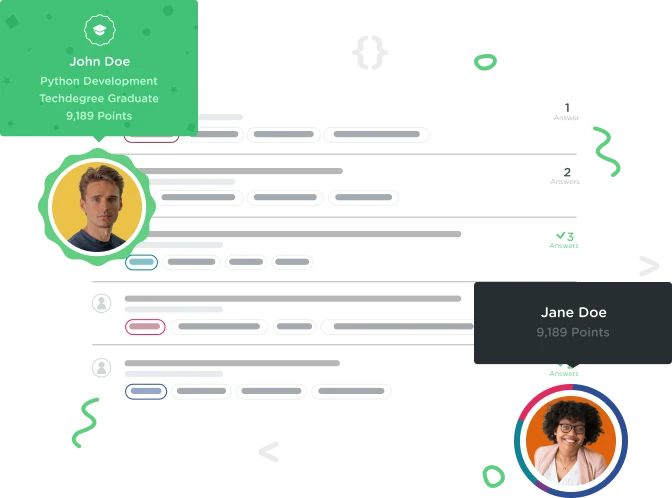
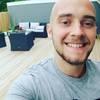
Wictor Niså
1,704 PointsChallenge task 1 of 2 JavaScript
I'm pretty confused and not sure how to complete this task, some insight in what im doing wrong would be really helpful, thanks!
```function max ( uno, dos ){ var tres = uno + dos; return tres if (uno > dos); }
max(5, 2); ```
function max ( uno, dos ){
var tres = uno + dos;
return tres if (uno > dos);
}
max(5, 2);
3 Answers

Alexander Solberg
14,350 PointsDon't worry, happens to me all the time :P the most frustrating part about learning how to code haha.
I solved the challenge like this
var max = function(a, b) {
if(a > b) {
return a;
} else {
return b;
}
}
Next you will have to call the function using an alert.
alert(max(12, 18));
I would recommend rewatching one or two videos about conditionals, and maybe some of the videos prior to the challenge, just to reinforce it conceptually.

Alexander Solberg
14,350 PointsThe max() function should take two parameters, we expect them to be numbers.
We want to return the higher number. In order to do this we need a conditional statement. If "a", we return a, else we return b.
This is all you need, you don't need to declare any other variable.
Have another go at it and I bet you will figure it out :)
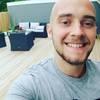
Wictor Niså
1,704 PointsI'm twisting my head around this one, sadly im only getting more confused by the minute.
I took your advice and got something like this
``` function max ( uno, dos ){ if (uno >= dos);{ return uno; } else if ( uno <= dos); return dos; }
var x = max(5, 2); ```
this doesn't work either, do you have an example you can give me or do would you recommend me to go back a few videos and revisit the function sections overall? Cheers for the help :)

Antti Lylander
9,686 Points//function takes two arguments, a and b
function max(a, b) {
// first, let's check if a is greater than b. If so, return a and the rest of the code in the function will not be executed as return ends it
if (a > b) {
return a;
}
//now, if a was not greater than b, return b (this will return b also when the numbers are equal but it's not the point here.)
//can use else here but it is not required
return b;
}
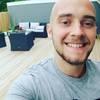
Wictor Niså
1,704 PointsThank you so much for the help and examples!