Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial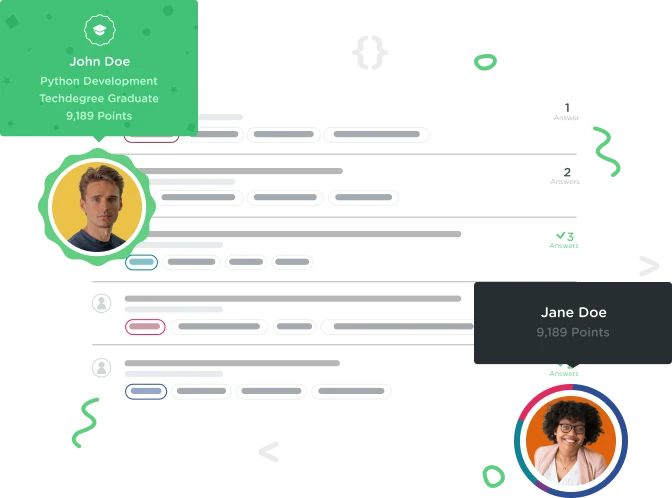

tangtai chen
4,844 PointsChallenge Task 1 of Enumeration and Options, can someone help?
In the editor below you have two objects - classes named Point and Robot. The Robot stores its location as a point instance and contains a move function.
The task of this challenge is to complete the implementation for move. Move takes a parameter of type Direction which is an enumeration listing the possible movement directions.
When you tell the robot to move up (by specifying Direction.Up as the argument), the y coordinate should increase by 1. Similarly moving down means the y coordinate decreases by 1, moving right means the x coordinate increases by 1 and finally left means x decreases by 1.
here is my code it seems alright in Xcode but I could spot the mistake, can someone plz help!
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
enum Direction {
case left
case right
case up
case down
}
class Robot {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: Direction) {
switch direction {
case .up: location.y += 1
case .down: location.y -= 1
case .right: location.x += 1
case .left: location.x -= 1
}
}
}
3 Answers
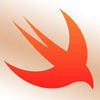
Jeff McDivitt
23,970 PointsIt look as though you altered the code that was given in the challenge
You have
func move(direction: Direction) {
switch direction {
case .up: location.y += 1
case .down: location.y -= 1
case .right: location.x += 1
case .left: location.x -= 1
}
}
}
should be
//Do not remove the underscore in front of direction
func move( _ direction: Direction) {
switch direction {
case .up: location.y += 1
case .down: location.y -= 1
case .right: location.x += 1
case .left: location.x -= 1
}
}
}
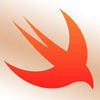
Jeff McDivitt
23,970 PointsAn underscore tells Swift that the parameter should have no label when called
Example
func add( _ x: Int, _ y: Int {
}
When you call the function you will not need to include the labels x and y
let myAdd = add(1,2)

tangtai chen
4,844 Pointsgot it thx

Aaryen Singh
1,688 PointsCool thx.
tangtai chen
4,844 Pointstangtai chen
4,844 Pointsthx it worked? what does the underscore do in the code anyways? in some challenges I removed the underscore of the code it worked