Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial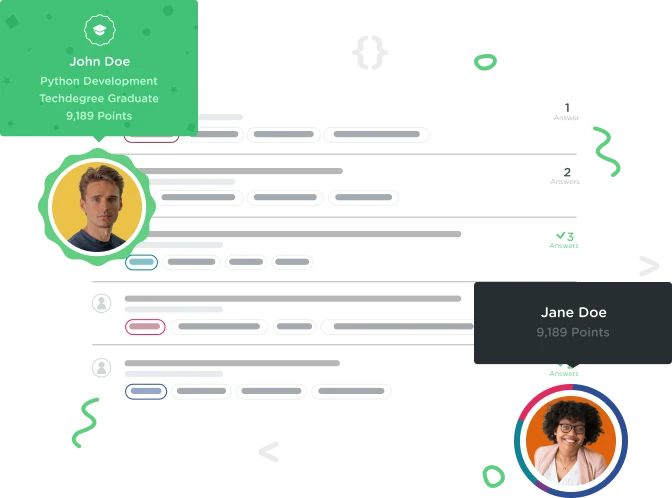

Anik Devaughn
7,751 PointsChallenge Task 1 : Using the map method on the authors array, create an array of full name strings, comprising the .....
Hi, I am stuck with this challenge and can't figure a way out, Please can someone explain what mistake i made and how to think when it comes solving this type of Challenges. Thanks in Advance !!! My Code: const authors = [ { firstName: "Beatrix", lastName: "Potter" }, { firstName: "Ann", lastName: "Martin" }, { firstName: "Beverly", lastName: "Cleary" }, { firstName: "Roald", lastName: "Dahl" }, { firstName: "Lewis", lastName: "Carroll" } ]; let fullAuthorNames;
// fullAuthorNames should be: ["Beatrix Potter", "Ann Martin", "Beverly Cleary", "Roald Dahl", "Lewis Carroll"] // Write your code below
fullAuthorNames = authors.reduce((fullAuthorNames, user) => { fullAuthorNames = user.firstName + " " + user.lastName; return fullAuthorNames; }, {});
console.log(fullAuthorNames);
const authors = [
{ firstName: "Beatrix", lastName: "Potter" },
{ firstName: "Ann", lastName: "Martin" },
{ firstName: "Beverly", lastName: "Cleary" },
{ firstName: "Roald", lastName: "Dahl" },
{ firstName: "Lewis", lastName: "Carroll" }
];
let fullAuthorNames;
// fullAuthorNames should be: ["Beatrix Potter", "Ann Martin", "Beverly Cleary", "Roald Dahl", "Lewis Carroll"]
// Write your code below
fullAuthorNames = authors.reduce((fullAuthorNames, user) => {
fullAuthorNames = user.firstName + " " + user.lastName;
return fullAuthorNames;
}, {});
console.log(fullAuthorNames);
2 Answers

Manish Giri
16,266 PointsYou need to use the .map()
method here, not .reduce()
.
Think of .map()
as mapping operation. You iterate over the source and transform the source from one type to another..Like in this example, you start with an array of objects. You use .map()
to iterate over the source and transform it into a new array, where each element is a string consisting of the full name. So you transform an array of objects to an array of strings. This is the common use case of .map()
.
.reduce()
is like a reducing operation, you iterate over an array of values and transform it into one single value.
Example -
var numbers = [1,2,3,4,5];
var sum = 0;
for(var i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
This is a normal for loop, you iterate over the numbers
array and find out the sum of all elements in the array.
In other words, you "reduce" the array to a single value. So you can rewrite the for
loop using a reduce()
-
var sum = numbers.reduce((a,b) => a + b);
Hope this helps.

Anik Devaughn
7,751 PointsHi again, Maybe I did not got your point or didnot understand , tried in different ways but still can't solve this challenge. Here what i tried with my code, Hope you acn help... const authors = [ { firstName: "Beatrix", lastName: "Potter" }, { firstName: "Ann", lastName: "Martin" }, { firstName: "Beverly", lastName: "Cleary" }, { firstName: "Roald", lastName: "Dahl" }, { firstName: "Lewis", lastName: "Carroll" } ]; let fullAuthorNames;
// fullAuthorNames should be: ["Beatrix Potter", "Ann Martin", "Beverly Cleary", "Roald Dahl", "Lewis Carroll"] // Write your code below
fullAuthorNames = authors .map((fullAuthorNames => ({fullAuthorNames. firstName}) + ({fullAuthorNames.lastName})));

Manish Giri
16,266 PointsYou're almost there, just need to clean up the code inside .map()
to follow the instructions the challenge asks -
fullAuthorNames = authors .map(author => `${ author.firstName} `${author.lastName}`);
Here, I'm using ES6 Template Strings.