Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial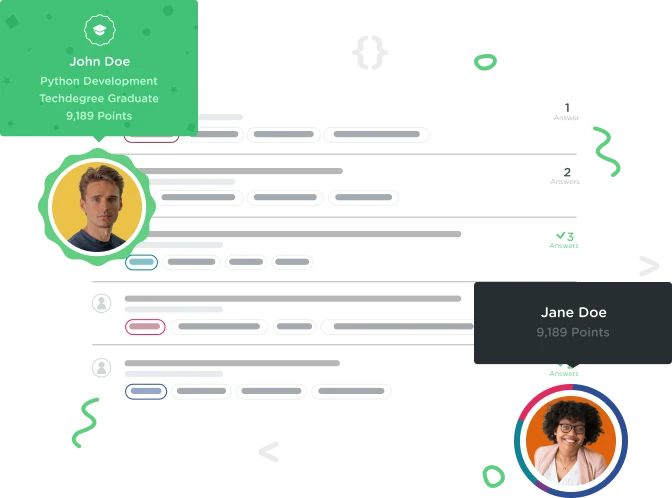

Brandon Oree
1,585 PointsChallenge Task 2 of 2, for looping (Obj-C)
I feel my code is mostly correct. I've even debugged this in Xcode, mathTotal starts with 5 and ends at 25. What am I doing wrong?
int mathTotal;
bool isComplete;
for (mathTotal = 5; mathTotal < 25; mathTotal++)
{
isComplete = FALSE;
}
1 Answer
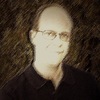
Jason Anders
Treehouse Moderator 145,858 PointsHey Brandon,
There are a few errors happening here:
For loops use temporary variables (I always use
i
) to iterate through the loop. The variablemathTotal
(an Integer) cannot be used in both the loop and as a variable outside of the loop. This is the variable that will hold the cumulative total as thefor loop
iterates and adds to the variable.Right now your loop will stop at 24 (because it is stating less than 25, which does not include 25). The challenge specifically asks you to keep the 25 in there, so you will need "less than OR equal to).
Your boolean value for
isComplete
needs to be outside of the loop block, as this will be assigned only when the loop is done.isComplete
needs to be set to "true" not "FALSE" after the loop.The incrementing code for
mathTotal
is missing. This needs to be inside of the loop, so with each iteration of the loop, the new value fori
gets added to the running total ofmathTotal
.
Below is the completed and corrected code for your reference. I hope it makes sense.
int mathTotal;
bool isComplete;
for (int i = 5; i <= 25; i++) {
mathTotal += i;
}
isComplete = true;
Keep Coding! :)
Brandon Oree
1,585 PointsBrandon Oree
1,585 PointsJason, I've looked through your code a few times and, though I know it's right, there are a few things that still puzzle me. I'll review the videos again, and continue studying your code, until I completely understand looping. Thank you for the assistance!
Jason Anders
Treehouse Moderator 145,858 PointsJason Anders
Treehouse Moderator 145,858 PointsI'll see if I can help a bit.
You declare the
int i = 5
. This tells the loop that you want to use the temporary variablei
to keep count, and you want it to start at 5. So, the start of the loop,i
= 5.Then the
i <= 25
checks the condition. In this case, it is checking to see ifi
is equal to or less than 25. It is, so the loop says to add 1 to the previous value ofi
and execute the code block.The code block executes and add the value of
i
to the variablemathTotal
. The loop now starts over withi
now equal to 6. It goes through again. Executes the code block and adds the new value ofi
to the existing value ofmathTotal
.It will do this until the value of
i
hits 26, which is now no longer less than or equal to 25. It's now that the code block will not execute, and will bounce out of the loop. The codeisComplete = true;
now runs (because it is outside of the loop and the loop has been completed).Reviewing is always a good idea, I often review and/or rewatch videos. It's best to have a good understanding before moving on.
Have a look at TutorialsPoint or just Google "for loop in objective-c". Honestly, you could also look at any JavaScript tutorials on
for loops
as they are pretty much exactly the same as Objective-C. Sometimes all one needs is an explanation given in a different way for it to click. :)Brandon Oree
1,585 PointsBrandon Oree
1,585 PointsI understand completely now. I think my confusion was stemming mostly from my unfamiliarity with the concept, but seeing your code and comparing what worked to what didn't, and why, helped. I hope I didn't bog down much of your time.
Jason Anders
Treehouse Moderator 145,858 PointsJason Anders
Treehouse Moderator 145,858 PointsNo worries!
Happy to help.