Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial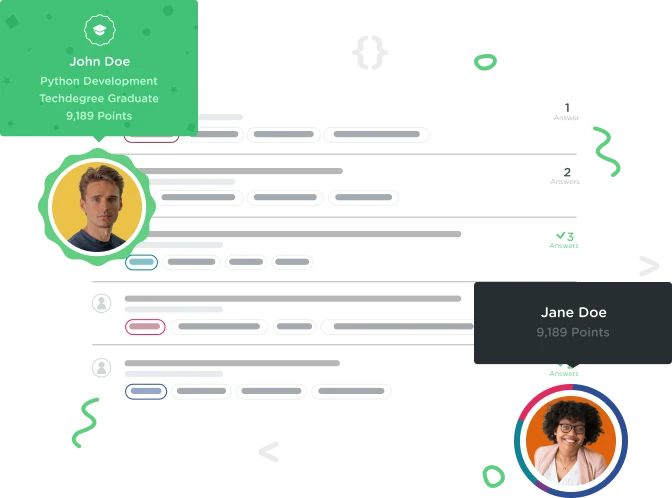
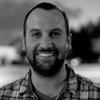
John Cotton
10,936 PointsChallenge Task 2 of 2 For this task, make WifiLamp adopt the ColorSwitchable protocol. In the implementation of the..
No preview section to debug compiler error...
I'm having trouble making this work.
// Declare protocol here
protocol ColorSwitchable{
func switchColor(color: Color)
}
enum LightState {
case On, Off
}
enum Color {
case RGB(Double, Double, Double, Double)
case HSB(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init() {
self.state = .On
self.color = .RGB(0,0,0,0)
}
func switchColor(color: Color) -> Color{
}
}
2 Answers

Travis McCormick
2,313 PointsHey John!
The challenge does not state that there will be a return type in the func
that was set as the stored property for the ColorSwitchable protocol
. So the first thing to do would be to remove the return ( -> Color ) from your func
.
Next, the func
in your code doesn't set the color of the lamp to a new color. We are just creating the Class
so there wont be a specific color here, that wont happen until an instance of this Class
is created. So the body of the func
would be self.color = color
Lastly, the func
would go before init
.
protocol ColorSwitchable {
func switchColor(color: Color)
}
enum LightState {
case On, Off
}
enum Color {
case RGB(Double, Double, Double, Double)
case HSB(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
func switchColor(color: Color) {
self.color = color
}
init() {
self.state = .On
self.color = .RGB(0,0,0,0)
}
}
I know this answer is a bit delayed but I hope that others may find it helpful if they need some help on this challenge as well.

Matthew Young
5,133 PointsAlthough you've defined a "switchColor" function in your class to conform to the ColorSwitchable protocol, you've defined it with a return type of "Color". Because of this, it isn't exactly the function listed in the protocol so your code still doesn't conform to the ColorSwitchable protocol.
To make it conform, you need to either get rid of the return type in the current "switchColor" function OR define another "switchColor" function without the return type (function names in Swift can be shared as long as the function signatures are different such as the parameters or return type).
(If you do decide to keep your current "switchColor" function as is, you'll need to return a type of "Color" or you'll get another error.)